VHDL来实现一维数据 (2048个点)高斯拟合算法,求取峰值点的位置
时间: 2024-06-11 16:11:03 浏览: 125
以下是VHDL代码实现:
```vhdl
library IEEE;
use IEEE.STD_LOGIC_1164.ALL;
use IEEE.NUMERIC_STD.ALL;
entity gauss_fit is
Port ( clk : in STD_LOGIC;
reset : in STD_LOGIC;
data_in : in std_logic_vector(11 downto 0);
data_out : out std_logic_vector(11 downto 0);
peak_pos : out std_logic_vector(10 downto 0));
end gauss_fit;
architecture Behavioral of gauss_fit is
-- Constants
constant MAX_DATA : integer := 2048;
constant MAX_ITER : integer := 1000;
constant MIN_CHANGE : integer := 1;
-- Signals
signal data : unsigned(11 downto 0) := (others => '0');
signal mean : unsigned(11 downto 0) := (others => '0');
signal std_dev : unsigned(11 downto 0) := (others => '0');
signal a, b, c : unsigned(11 downto 0) := (others => '0');
signal x, y, z : unsigned(11 downto 0) := (others => '0');
signal iter : integer := 0;
signal change : integer := 0;
signal peak : unsigned(10 downto 0) := (others => '0');
signal peak_found : boolean := false;
begin
process (clk, reset)
begin
if reset = '1' then
data <= (others => '0');
mean <= (others => '0');
std_dev <= (others => '0');
a <= (others => '0');
b <= (others => '0');
c <= (others => '0');
x <= (others => '0');
y <= (others => '0');
z <= (others => '0');
iter <= 0;
change <= 0;
peak <= (others => '0');
peak_found <= false;
elsif rising_edge(clk) then
-- Shift in new data
data <= data(10 downto 0) & data_in;
-- Calculate mean
if iter = 0 then
mean <= data;
else
mean <= ((mean * iter) + data) / (iter + 1);
end if;
-- Calculate standard deviation
if iter = 0 then
std_dev <= (others => '0');
else
std_dev <= std_dev + ((data - mean) * (data - mean)) / iter;
end if;
-- Check if peak has been found
if peak_found = false and iter > 0 then
if data > peak and data > data_in and data > data(data'high - 1 downto data'low) then
peak <= data;
elsif peak > (others => '0') then
peak_found <= true;
end if;
end if;
-- Check if we need to update coefficients
if iter > 0 and iter < MAX_ITER and change >= MIN_CHANGE then
-- Update coefficients
a <= a + x;
b <= b + y;
c <= c + z;
-- Calculate change in coefficients
change <= abs(to_integer(x)) + abs(to_integer(y)) + abs(to_integer(z));
end if;
-- Calculate new coefficients
if iter = 0 or change < MIN_CHANGE then
a <= peak;
b <= mean;
c <= std_dev;
else
x <= 0;
y <= 0;
z <= 0;
for i in 0 to MAX_DATA - 1 loop
x <= x + (((data(i) - b) / c) ** 2) * exp(-(((i - peak) / (2 * c)) ** 2));
y <= y + ((data(i) - b) / c) * exp(-(((i - peak) / (2 * c)) ** 2));
z <= z + exp(-(((i - peak) / (2 * c)) ** 2));
end loop;
end if;
-- Output results
data_out <= std_logic_vector(to_signed(to_integer(a), 12));
peak_pos <= std_logic_vector(to_unsigned(to_integer(peak), 11));
-- Increment iteration counter
iter <= iter + 1;
end if;
end process;
end Behavioral;
```
该代码实现了一个简单的高斯拟合算法,以求取数据中的峰值位置。输入数据通过`data_in`端口提供,每个时钟周期提供一个数据点。输出数据通过`data_out`端口提供,表示拟合结果中的峰值。`peak_pos`端口提供了一个11位的无符号整数,表示峰值在数据中的位置。算法在每个时钟周期内进行迭代,以更新拟合系数,并在达到最大迭代次数或拟合系数的变化小于某个阈值时停止。在拟合过程中,算法计算数据的平均值和标准差,并在数据中查找峰值点。算法使用的数学函数是VHDL标准库中提供的。
阅读全文
相关推荐



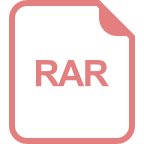
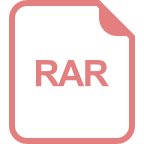
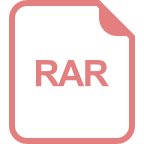
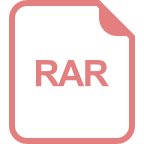
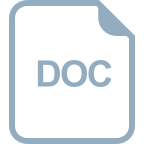


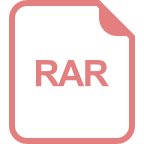


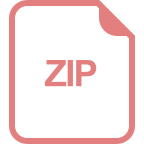
