用java生成股票经纪人要在一群人(n 个人的编号为0~n-1)中散布一个传言,传言只在认识的人中间传递。题目中给出了人与人的认识关系以及传言在某两个认识的人中传递所需要的时间。编写程序求出以哪个人为起点可以在耗时最短的情况下让所有人收到消息。 例如,n=4(人数),m=4(边数),4 条边如下。 0 1 2 0 2 5 0 3 1 2 3 3 输出:3
时间: 2024-02-11 11:09:43 浏览: 47
好的,这是一个经典的图论问题,可以使用 Dijkstra 算法或者 Floyd 算法来解决。以下是一个使用 Dijkstra 算法的示例代码:
```java
import java.util.*;
public class Main {
static class Edge {
int from;
int to;
int weight;
public Edge(int from, int to, int weight) {
this.from = from;
this.to = to;
this.weight = weight;
}
}
static class Node implements Comparable<Node> {
int id;
int distance;
public Node(int id, int distance) {
this.id = id;
this.distance = distance;
}
@Override
public int compareTo(Node o) {
return Integer.compare(this.distance, o.distance);
}
}
static List<List<Edge>> graph = new ArrayList<>();
static int[] distances;
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int n = scanner.nextInt();
int m = scanner.nextInt();
for (int i = 0; i < n; i++) {
graph.add(new ArrayList<>());
}
for (int i = 0; i < m; i++) {
int u = scanner.nextInt();
int v = scanner.nextInt();
int w = scanner.nextInt();
graph.get(u).add(new Edge(u, v, w));
graph.get(v).add(new Edge(v, u, w));
}
int minDistance = Integer.MAX_VALUE;
int minNode = -1;
for (int i = 0; i < n; i++) {
distances = new int[n];
Arrays.fill(distances, Integer.MAX_VALUE);
distances[i] = 0;
PriorityQueue<Node> queue = new PriorityQueue<>();
queue.add(new Node(i, 0));
while (!queue.isEmpty()) {
Node node = queue.poll();
if (node.distance != distances[node.id]) {
continue;
}
for (Edge edge : graph.get(node.id)) {
if (distances[edge.to] > distances[edge.from] + edge.weight) {
distances[edge.to] = distances[edge.from] + edge.weight;
queue.add(new Node(edge.to, distances[edge.to]));
}
}
}
int maxDistance = 0;
for (int j = 0; j < n; j++) {
if (distances[j] == Integer.MAX_VALUE) {
maxDistance = Integer.MAX_VALUE;
break;
}
maxDistance = Math.max(maxDistance, distances[j]);
}
if (maxDistance < minDistance) {
minDistance = maxDistance;
minNode = i;
}
}
System.out.println(minNode);
}
}
```
在这个示例代码中,我们首先读入节点数量和边的数量,然后建立一个邻接表表示图。接着我们遍历所有节点,以每个节点为起点使用 Dijkstra 算法计算最短路径。最后我们找到所有最短路径中的最大值,即为所有节点都收到消息所需的最小时间。最小时间最短的起点即为答案。
阅读全文
相关推荐
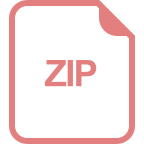
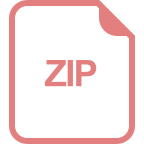












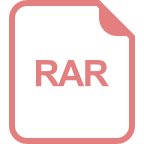
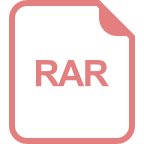
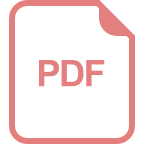