使用uniapp写一个瀑布流
时间: 2023-12-03 19:01:46 浏览: 30
好的,下面是使用uniapp实现瀑布流的步骤:
1. 在uniapp项目中创建一个页面,命名为“waterfall”,并在pages.json中配置路由。
2. 在waterfall.vue中,先引入所需的组件和样式:
```
<template>
<view class="waterfall">
<view class="waterfall-list">
<view class="waterfall-item" v-for="(item, index) in list" :key="index">
<image class="waterfall-img" :src="item.imgUrl"></image>
<view class="waterfall-info">{{ item.title }}</view>
</view>
</view>
</view>
</template>
<style>
.waterfall {
padding: 10rpx;
}
.waterfall-list {
display: flex;
flex-wrap: wrap;
justify-content: space-between;
}
.waterfall-item {
width: 48%;
margin-bottom: 20rpx;
border-radius: 10rpx;
overflow: hidden;
box-shadow: 0 2rpx 10rpx rgba(0, 0, 0, 0.1);
}
.waterfall-img {
width: 100%;
height: 0;
padding-bottom: 100%;
display: block;
}
.waterfall-info {
padding: 10rpx;
font-size: 28rpx;
color: #333;
}
</style>
```
3. 在script标签中,定义data和methods:
```
<script>
export default {
data() {
return {
list: [
{
title: '图片1',
imgUrl: 'https://picsum.photos/id/1051/600/800'
},
{
title: '图片2',
imgUrl: 'https://picsum.photos/id/1052/600/800'
},
{
title: '图片3',
imgUrl: 'https://picsum.photos/id/1053/600/800'
},
{
title: '图片4',
imgUrl: 'https://picsum.photos/id/1054/600/800'
},
{
title: '图片5',
imgUrl: 'https://picsum.photos/id/1055/600/800'
},
{
title: '图片6',
imgUrl: 'https://picsum.photos/id/1056/600/800'
}
]
}
}
}
</script>
```
4. 在mounted生命周期钩子中,计算每个item的高度,并按照比例设置图片高度:
```
<script>
export default {
data() {
return {
list: [
{
title: '图片1',
imgUrl: 'https://picsum.photos/id/1051/600/800'
},
{
title: '图片2',
imgUrl: 'https://picsum.photos/id/1052/600/800'
},
{
title: '图片3',
imgUrl: 'https://picsum.photos/id/1053/600/800'
},
{
title: '图片4',
imgUrl: 'https://picsum.photos/id/1054/600/800'
},
{
title: '图片5',
imgUrl: 'https://picsum.photos/id/1055/600/800'
},
{
title: '图片6',
imgUrl: 'https://picsum.photos/id/1056/600/800'
}
]
}
},
mounted() {
const query = uni.createSelectorQuery().in(this)
query.selectAll('.waterfall-img').boundingClientRect(rects => {
rects.forEach(rect => {
const width = rect.width
const height = rect.height
const ratio = height / width
rect.dataset.ratio = ratio
})
this.setImageHeight()
}).exec()
},
methods: {
setImageHeight() {
this.list.forEach(item => {
uni.getImageInfo({
src: item.imgUrl,
success: res => {
const width = res.width
const ratio = this.$refs['waterfall-list'].offsetWidth / width
item.imgHeight = res.height * ratio
}
})
})
}
}
}
</script>
```
5. 在模板中,使用v-bind动态绑定图片高度:
```
<template>
<view class="waterfall">
<view class="waterfall-list" ref="waterfall-list">
<view class="waterfall-item" v-for="(item, index) in list" :key="index">
<image class="waterfall-img" :src="item.imgUrl" :style="{ height: item.imgHeight + 'px' }"></image>
<view class="waterfall-info">{{ item.title }}</view>
</view>
</view>
</view>
</template>
```
6. 最后,可以在App.vue中导航到waterfall页面进行预览。
这样就完成了使用uniapp实现瀑布流的步骤。
相关推荐
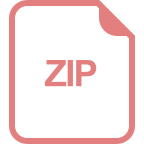














