np.cumsum(rate)
时间: 2024-04-25 16:24:25 浏览: 128
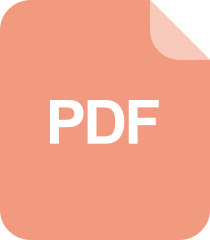
python numpy中cumsum的用法详解

`np.cumsum(rate)` 是使用 NumPy 库中的 `cumsum()` 函数对给定数组 `rate` 进行累积求和操作。
`cumsum()` 函数将数组中的元素逐个累加起来,并返回一个新的数组,其中每个元素是原始数组对应位置及之前所有元素的累积和。
示例:
```python
import numpy as np
rate = [1, 2, 3, 4, 5]
cumulative_sum = np.cumsum(rate)
print(cumulative_sum) # [ 1 3 6 10 15]
```
在这个示例中,给定的数组 `rate` 是 `[1, 2, 3, 4, 5]`。通过调用 `np.cumsum(rate)`,得到了一个新的数组 `cumulative_sum`,其中每个元素是原始数组 `rate` 对应位置及之前所有元素的累积和。结果为 `[1, 3, 6, 10, 15]`。
阅读全文
相关推荐
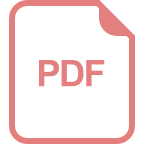
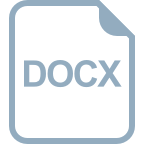
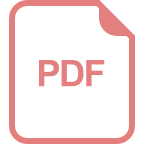
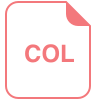
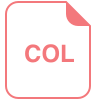






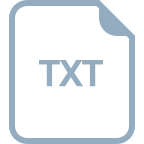
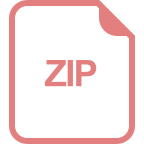
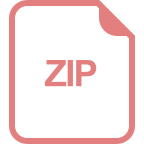
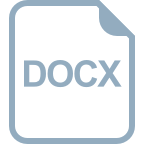
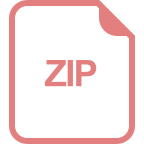
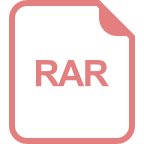