python在5000*4000二维矩阵中求20*20二维移动窗口内极大值的坐标
时间: 2023-04-02 11:04:54 浏览: 50
可以使用numpy库中的函数实现,具体代码如下:
import numpy as np
# 生成一个500*400的随机矩阵
matrix = np.random.rand(500, 400)
# 定义移动窗口的大小
window_size = 20
# 使用maximum_filter函数求出每个窗口内的最大值
max_matrix = np.maximum_filter(matrix, size=(window_size, window_size))
# 使用argwhere函数找到最大值的坐标
max_coords = np.argwhere(matrix == max_matrix)
# 输出第一个最大值的坐标
print(max_coords[])
相关问题
python在5000*4000二维矩阵中求每个20*20二维移动窗口内极大值的坐标
可以使用numpy库中的stride_tricks函数来实现这个功能。具体步骤如下:
1. 将二维矩阵转换为一维数组,方便后续操作。
2. 使用stride_tricks函数将一维数组转换为二维数组,每个子数组的大小为20*20。
3. 对每个子数组求最大值的坐标。
4. 将每个子数组的最大值坐标转换为在原始二维矩阵中的坐标。
代码示例:
import numpy as np
# 生成一个500*400的随机二维矩阵
matrix = np.random.rand(500, 400)
# 定义移动窗口大小
window_size = 20
# 将二维矩阵转换为一维数组
flat_matrix = matrix.ravel()
# 使用stride_tricks函数将一维数组转换为二维数组
shape = (matrix.shape[]-window_size+1, matrix.shape[1]-window_size+1, window_size, window_size)
strides = (matrix.strides[], matrix.strides[1], matrix.strides[], matrix.strides[1])
sub_matrices = np.lib.stride_tricks.as_strided(flat_matrix, shape=shape, strides=strides)
# 对每个子数组求最大值的坐标
max_indices = np.unravel_index(np.argmax(sub_matrices, axis=(2,3)), shape[:2])
# 将每个子数组的最大值坐标转换为在原始二维矩阵中的坐标
max_indices = (max_indices[]+window_size//2, max_indices[1]+window_size//2)
print(max_indices)
python二维矩阵求二维移动窗口内极大值的坐标
可以使用numpy库中的函数numpy.lib.stride_tricks.as_strided来实现二维移动窗口的操作,具体实现可以参考以下代码:
import numpy as np
def max_in_window(matrix, window_size):
# 计算窗口的步长
stride = matrix.strides
stride = (stride[], stride[1], stride[], stride[1])
# 利用as_strided函数生成移动窗口的视图
window_view = np.lib.stride_tricks.as_strided(matrix, shape=(
matrix.shape[] - window_size + 1, matrix.shape[1] - window_size + 1, window_size, window_size), strides=stride)
# 计算每个窗口内的最大值的坐标
max_index = np.unravel_index(np.argmax(window_view, axis=(2, 3)), (window_view.shape[], window_view.shape[1]))
# 将坐标转换为在原矩阵中的位置
max_index = np.array(max_index).T
max_index[:, ] += window_size // 2
max_index[:, 1] += window_size // 2
return max_index
# 测试代码
matrix = np.random.randint(, 10, size=(5, 5))
print(matrix)
print(max_in_window(matrix, 3))
相关推荐
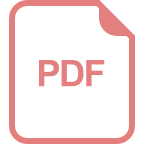
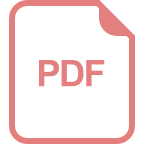
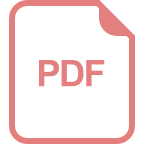












