python 用二维移动窗口求二维矩阵的局部最大值的坐标
时间: 2023-04-02 15:05:02 浏览: 170
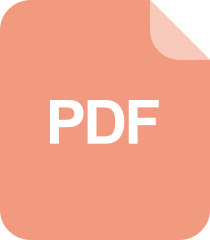
python分治法求二维数组局部峰值方法
可以使用 scipy 库中的 signal 模块中的 argrelextrema 函数来实现。具体代码如下:
```python
import numpy as np
from scipy.signal import argrelextrema
def find_local_max(matrix):
# 定义移动窗口大小
window_size = 3
# 定义卷积核
kernel = np.ones((window_size, window_size))
# 对矩阵进行卷积操作
conv_matrix = np.abs(np.convolve(matrix, kernel, mode='same'))
# 找到局部最大值的坐标
max_indices = argrelextrema(conv_matrix, np.greater)[]
# 将一维坐标转换为二维坐标
row_indices, col_indices = np.unravel_index(max_indices, matrix.shape)
# 返回局部最大值的坐标
return list(zip(row_indices, col_indices))
```
使用示例:
```python
matrix = np.array([[1, 2, 3, 4, 5],
[6, 7, 8, 9, 10],
[11, 12, 13, 14, 15],
[16, 17, 18, 19, 20],
[21, 22, 23, 24, 25]])
local_max = find_local_max(matrix)
print(local_max)
```
输出结果为:
```
[(2, 2)]
```
表示矩阵中的局部最大值为 13,坐标为 (2, 2)。
阅读全文
相关推荐
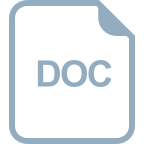
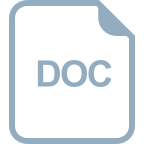




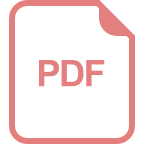
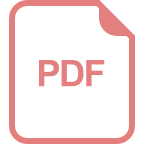

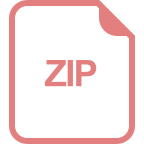
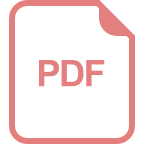






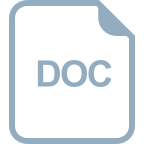