用c++定义函数createFile创建一个文本文件person.txt,将n个Person对象写入文件,再定义函数readFile将文件中的信息读出显示在屏幕上。
时间: 2024-03-10 17:47:20 浏览: 18
好的,以下是一个C++实现:
```c++
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
class Person {
public:
string name;
int age;
};
void createFile(int n) {
ofstream fout("person.txt");
for (int i = 0; i < n; i++) {
Person person;
cout << "请输入第" << i + 1 << "个人的姓名:";
cin >> person.name;
cout << "请输入第" << i + 1 << "个人的年龄:";
cin >> person.age;
fout << person.name << " " << person.age << endl;
}
fout.close();
}
void readFile() {
ifstream fin("person.txt");
string line;
while (getline(fin, line)) {
Person person;
sscanf(line.c_str(), "%s %d", person.name.c_str(), &person.age);
cout << "姓名:" << person.name << ",年龄:" << person.age << endl;
}
fin.close();
}
int main() {
int n;
cout << "请输入要写入文件中的Person对象数量:";
cin >> n;
createFile(n);
cout << "成功将" << n << "个Person对象写入文件!" << endl;
cout << "接下来读取文件中的信息:" << endl;
readFile();
return 0;
}
```
使用时,首先调用`createFile(n)`函数将`n`个`Person`对象写入文件。写入成功后,再调用`readFile()`函数将文件中的信息读出并显示在屏幕上。在`createFile(n)`函数中,我们使用`ofstream`类打开文件,并使用`<<`运算符将每个`Person`对象的姓名和年龄写入文件。在`readFile()`函数中,我们使用`ifstream`类打开文件,并使用`getline()`函数逐行读取文件内容。然后,我们使用`sscanf()`函数将每行字符串按空格分隔成姓名和年龄两个部分,并将它们存储在一个`Person`对象中。最后,我们打印出这个`Person`对象的姓名和年龄。
相关推荐
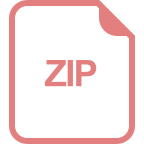
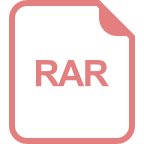
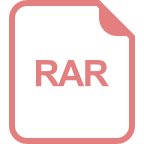





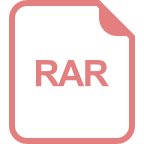
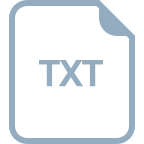
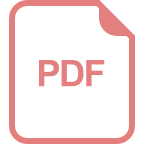
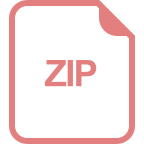
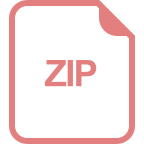
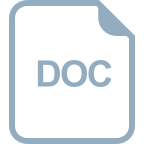
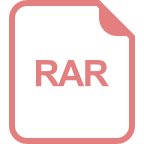
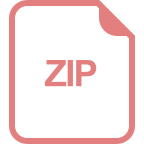