此代码有什么问题import cv2 import numpy as np import matplotlib.pyplot as plt img = cv2.imread(r'E:\\postgraduate\\three\\DIP3E_Original_Images_CH03\\3.14.tif',0) def bit_plane_slicing(src,z): height, width= src.shape dst = np.zeros((height, width), np.uint8) cv2.normalize(img, dst=dst, alpha=0, beta=1.0) for i in range(0, height): for j in range(0, width): pixel = format(src[i,j], '08b') if pixel[8-z] == '0': dst[i, j] = 0 else: dst[i, j] = 255 return dst img1 = bit_plane_slicing(img,8) img2 = bit_plane_slicing(img,7) img3 = bit_plane_slicing(img,6) img4 = bit_plane_slicing(img,5) image1 = img1*128 + img2*64 image2 = img1*128 + img2*64 + img3*32 image3 = img1*128 + img2*64 + img3*32 + img4*16 plt.figure(figsize=(100,100)) plt.subplot(131) plt.imshow(image1,cmap='gray') plt.axis('off') plt.subplot(132) plt.imshow(image2,cmap='gray') plt.axis('off') plt.subplot(133) plt.imshow(image3,cmap='gray') plt.axis('off') plt.show()
时间: 2024-03-17 20:41:37 浏览: 51
There are a few issues with this code:
1. The `bit_plane_slicing` function takes in a parameter `src`, but the code inside the function uses `img` instead. This will cause an error as `img` is not defined inside the function.
2. The `cv2.normalize` function is being used incorrectly. It should be `cv2.normalize(src, dst, alpha=0, beta=1.0, norm_type=cv2.NORM_MINMAX)`.
3. The `image1`, `image2`, and `image3` variables are being calculated incorrectly. The correct calculations should be:
```
image1 = img1*(2**7) + img2*(2**6)
image2 = img1*(2**7) + img2*(2**6) + img3*(2**5)
image3 = img1*(2**7) + img2*(2**6) + img3*(2**5) + img4*(2**4)
```
Here's the corrected code:
```
import cv2
import numpy as np
import matplotlib.pyplot as plt
img = cv2.imread(r'E:\\postgraduate\\three\\DIP3E_Original_Images_CH03\\3.14.tif',0)
def bit_plane_slicing(src,z):
height, width = src.shape
dst = np.zeros((height, width), np.uint8)
cv2.normalize(src, dst, alpha=0, beta=1.0, norm_type=cv2.NORM_MINMAX)
for i in range(0, height):
for j in range(0, width):
pixel = format(src[i,j], '08b')
if pixel[8-z] == '0':
dst[i, j] = 0
else:
dst[i, j] = 255
return dst
img1 = bit_plane_slicing(img,8)
img2 = bit_plane_slicing(img,7)
img3 = bit_plane_slicing(img,6)
img4 = bit_plane_slicing(img,5)
image1 = img1*(2**7) + img2*(2**6)
image2 = img1*(2**7) + img2*(2**6) + img3*(2**5)
image3 = img1*(2**7) + img2*(2**6) + img3*(2**5) + img4*(2**4)
plt.figure(figsize=(100,100))
plt.subplot(131)
plt.imshow(image1,cmap='gray')
plt.axis('off')
plt.subplot(132)
plt.imshow(image2,cmap='gray')
plt.axis('off')
plt.subplot(133)
plt.imshow(image3,cmap='gray')
plt.axis('off')
plt.show()
```
阅读全文
相关推荐








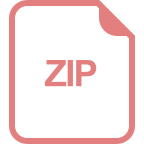
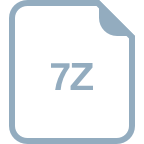
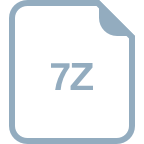
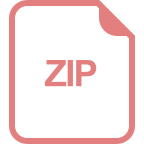