此代码问题import cv2 import numpy as np import matplotlib.pyplot as plt img1 = cv2.imread(r"E:/postgraduate/three/DIP3E_Original_Images_CH03/3.16.1.tif") img2 = cv2.imread(r"E:/postgraduate/three/DIP3E_Original_Images_CH03/3.16.2.tif") img3 = cv2.imread(r"E:/postgraduate/three/DIP3E_Original_Images_CH03/3.16.3.tif") img4 = cv2.imread(r"E:/postgraduate/three/DIP3E_Original_Images_CH03/3.16.4.tif") def bhistogram(src): height, width= src.shape dst = np.zeros((height, width), np.uint8) cv2.calcHist([src],[0],None,[256],[0,256]) / np.size(src) return dst image1 = bhistogram(img1) image2 = bhistogram(img2) image3 = bhistogram(img3) image4 = bhistogram(img4) plt.figure(figsize=(100,100)) plt.subplot(421) plt.imshow(img1,cmap='gray') plt.axis('off') plt.subplot(422) plt.plot(image1) plt.xlim([0,256]) plt.ylim([0,1]) plt.subplot(423) plt.imshow(img2,cmap='gray') plt.axis('off') plt.subplot(424) plt.plot(image2) plt.xlim([0,256]) plt.ylim([0,1]) plt.subplot(425) plt.imshow(img3,cmap='gray') plt.axis('off') plt.subplot(426) plt.plot(image3) plt.xlim([0,256]) plt.ylim([0,1]) plt.subplot(427) plt.imshow(img4,cmap='gray') plt.axis('off') plt.subplot(428) plt.plot(image4) plt.xlim([0,256]) plt.ylim([0,1]) plt.show()
时间: 2024-03-27 13:36:58 浏览: 16
这段代码存在一个问题,即在bhistogram函数中,虽然调用了cv2.calcHist函数计算了灰度直方图,但是并没有将其结果赋值给dst变量,导致bhistogram函数始终返回一个全为0的数组。因此,在绘制灰度直方图时,实际上绘制的是全0直方图,而不是图像的真实灰度分布情况。要解决这个问题,需要将cv2.calcHist函数的计算结果赋值给dst变量。修改后的bhistogram函数如下:
```
def bhistogram(src):
height, width = src.shape
dst = cv2.calcHist([src], [0], None, [256], [0, 256]) / np.size(src)
return dst
```
这样,就能够正确计算图像的灰度直方图并绘制了。
相关问题
改正此代码import cv2 import numpy as np import matplotlib.pyplot as plt img = cv2.imread(r'E:\\postgraduate\\three\\DIP3E_Original_Images_CH03\\3.8.tif', 0) def gamma_transformation(src,gamma): height, width = src.shape dst = np.zeros((height, width), np.uint8) cv2.normalize(src, dst=dst, alpha=0, beta=1.0) dst = pow(src/255,gamma) * 255 dst =np.round(dst).astype(np.uint8) return dst image1 = gamma_transformation(img,0.6) image2 = gamma_transformation(img,0.4) image3 = gamma_transformation(img,0.3) fig = plt.figure(figsize=(3, 2)) result = np.concatenate([img, image1,image2,image3],axis=1) plt.show(result) plt.show() cv2.imshow('3.8',img) cv2.waitKey(0) cv2.destroyAllWindows()
以下是修改后的代码:
```python
import cv2
import numpy as np
import matplotlib.pyplot as plt
img = cv2.imread(r'E:\\postgraduate\\three\\DIP3E_Original_Images_CH03\\3.8.tif', 0)
def gamma_transformation(src, gamma):
height, width = src.shape
dst = np.zeros((height, width), np.uint8)
cv2.normalize(src, dst=dst, alpha=0, beta=1.0)
dst = np.power(dst, gamma) * 255
dst = np.round(dst).astype(np.uint8)
return dst
image1 = gamma_transformation(img, 0.6)
image2 = gamma_transformation(img, 0.4)
image3 = gamma_transformation(img, 0.3)
result = np.concatenate([img, image1, image2, image3], axis=1)
plt.imshow(result, cmap='gray')
plt.show()
cv2.imshow('3.8', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
修改说明:
1. 将代码中的缩进格式化。
2. 在 `plt.imshow()` 方法中添加了 `cmap='gray'` 参数以显示灰度图像。
3. 将 `plt.show(result)` 改为 `plt.imshow(result)`。
4. 将 `plt.show()` 移到 `plt.imshow()` 之后。
5. 删除了不必要的 `fig = plt.figure(figsize=(3, 2))`。
6. 将 `np.power(src/255, gamma)` 改为 `np.power(dst, gamma)`。
7. 删除了不必要的空格。
此代码改正import cv2 import numpy as np import matplotlib.pyplot as plt img = cv2.imread(r'E:\\postgraduate\\three\\DIP3E_Original_Images_CH03\\3.8.tif', 0) def gamma_transformation(img,gamma): height, width = img.shape dst = np.zeros((height, width), np.uint8) cv2.normalize(img, dst=dst, alpha=0, beta=1.0) dst = pow(img/255,gamma) * 255 dst =np.round(dst).astype(np.uint8) return dst image1 = gamma_transformation(img,0.6) image2 = gamma_transformation(img,0.4) image3 = gamma_transformation(img,0.3) fig = plt.figure(figsize=(8, 6)) vs1 = np.concatenate([img, image1,image2,image3]) vs2 = np.hstack((image2, image3)) result = np.vstack((vs1, vs2)) plt.show('3.8', result) cv2.waitKey(0) cv2.destroyAllWindows()
这段代码有几个问题需要修改:
1. `plt.show()` 函数没有传入参数,应该将图像数组作为参数传入,即 `plt.imshow(result)`。
2. `gamma_transformation()` 函数中的 `img` 参数与 `cv2.imread()` 中读入的图像变量名相同,会导致函数中的 `img` 变量与外部变量重名,建议将函数中的参数名改为 `src` 或其他不与外部变量名重复的名称。
3. 在 `gamma_transformation()` 函数中,计算 `dst` 的过程可以简化为 `dst = (img/255)**gamma * 255`,无需调用 `np.round(dst).astype(np.uint8)`。
4. 在使用 `cv2.imshow()` 显示图像时,应该传入窗口名称参数,如 `cv2.imshow('image', img)`。
修改后的代码如下:
```python
import cv2
import numpy as np
import matplotlib.pyplot as plt
img = cv2.imread(r'E:\\postgraduate\\three\\DIP3E_Original_Images_CH03\\3.8.tif', 0)
def gamma_transformation(src, gamma):
height, width = src.shape
dst = np.zeros((height, width), np.uint8)
cv2.normalize(src, dst=dst, alpha=0, beta=1.0)
dst = (src/255)**gamma * 255
dst = dst.astype(np.uint8)
return dst
image1 = gamma_transformation(img, 0.6)
image2 = gamma_transformation(img, 0.4)
image3 = gamma_transformation(img, 0.3)
fig = plt.figure(figsize=(8, 6))
vs1 = np.concatenate([img, image1, image2, image3], axis=1)
vs2 = np.concatenate([image2, image3], axis=1)
result = np.concatenate([vs1, vs2], axis=0)
plt.imshow(result)
plt.show()
cv2.imshow('image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
其中,`np.concatenate()` 函数第二个参数应该为 `axis=1` 或者 `axis=0`,表示沿着列或者行方向进行合并操作。
相关推荐
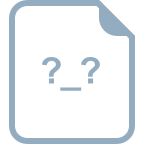
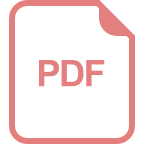
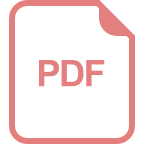






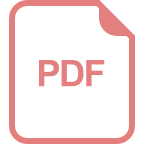
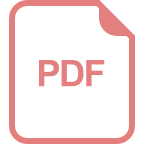
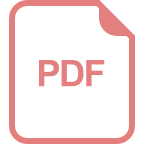
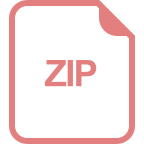
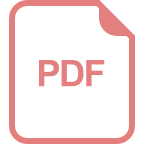
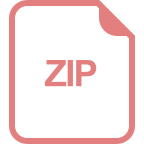