修改此代码import cv2 import numpy as np import matplotlib.pyplot as plt img = cv2.imread(r'E:\\postgraduate\\three\\DIP3E_Original_Images_CH03\\3.10.tif', 0) def contrast_strech(src,r1,s1,r2,s2): height, width = src.shape dst = np.zeros((height, width), np.uint8) r_min, r_max = np.min(img),np.max(img) for i in range(0, height): for j in range(0, width): dst[i, j] = (255 - 0) / (r_max - r_min) * ((img(i, j) - r_min)) return dst image1 = contrast_strech(img, np.min(img), 0, np.max(img), 255) image2 = contrast_strech(img, (np.min(img) + np.max(img)) / 2, 0, (np.min(img) + np.max(img)) / 2, 255) plt.figure(figsize=(100,100)) plt.subplot(131) plt.imshow(img,cmap='gray') plt.axis('off') plt.subplot(132) plt.imshow(image1,cmap='gray') plt.axis('off') plt.subplot(133) plt.imshow(image2,cmap='gray') plt.axis('off') plt.show()
时间: 2024-03-09 21:47:47 浏览: 60
这段代码存在一些错误,我帮你指出并修改:
1. 在函数 contrast_strech 中,第一行应该为 `height, width = src.shape` 而不是 `height, width = img.shape`,因为函数的参数为 `src`。
2. 在函数 contrast_strech 中,第四行应该为 `r_min, r_max = np.min(src), np.max(src)` 而不是 `r_min, r_max = np.min(img), np.max(img)`,因为函数的参数为 `src`。
3. 在函数 contrast_strech 中,第六行应该为 `dst[i, j] = (s2 - s1) / (r2 - r1) * (src[i, j] - r1) + s1`,而不是 `dst[i, j] = (255 - 0) / (r_max - r_min) * ((img(i, j) - r_min))`,因为你要进行对比度拉伸,不是简单的线性变换。
4. 在函数调用的时候,第二个对比度拉伸的函数调用应该为 `image2 = contrast_strech(img, (np.min(img) + np.max(img)) / 2, 0, (np.min(img) + np.max(img)) / 2, 255)` 而不是 `image2 = contrast_strech(img, np.min(img), 0, np.max(img), 255)`,因为这里使用的是对比度拉伸,而不是简单的线性变换。
修改后的代码如下:
```python
import cv2
import numpy as np
import matplotlib.pyplot as plt
img = cv2.imread(r'E:\\postgraduate\\three\\DIP3E_Original_Images_CH03\\3.10.tif', 0)
def contrast_strech(src, r1, s1, r2, s2):
height, width = src.shape
dst = np.zeros((height, width), np.uint8)
r_min, r_max = np.min(src), np.max(src)
for i in range(0, height):
for j in range(0, width):
if src[i, j] < r1:
dst[i, j] = s1 / r1 * src[i, j]
elif src[i, j] < r2:
dst[i, j] = (s2 - s1) / (r2 - r1) * (src[i, j] - r1) + s1
else:
dst[i, j] = (255 - s2) / (255 - r2) * (src[i, j] - r2) + s2
return dst
image1 = contrast_strech(img, np.min(img), 0, np.max(img), 255)
image2 = contrast_strech(img, (np.min(img) + np.max(img)) / 2, 0, (np.min(img) + np.max(img)) / 2, 255)
plt.figure(figsize=(15, 5))
plt.subplot(131)
plt.imshow(img, cmap='gray')
plt.axis('off')
plt.subplot(132)
plt.imshow(image1, cmap='gray')
plt.axis('off')
plt.subplot(133)
plt.imshow(image2, cmap='gray')
plt.axis('off')
plt.show()
```
希望对你有帮助!
阅读全文
相关推荐
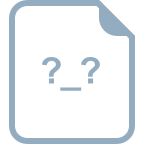








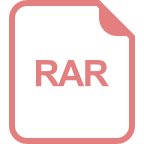
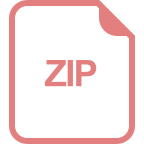