dijkstra算法c语言邻接表
时间: 2023-10-20 16:02:34 浏览: 54
Dijkstra算法的C语言邻接表实现可以参考以下代码:
```c
#include <stdio.h>
#include <stdbool.h>
#define MAX 1000
#define INF 9999
typedef struct Node {
int vertex;
int weight;
struct Node* next;
} Node;
typedef struct Graph {
Node* adjList[MAX];
int V;
int E;
} Graph;
Graph* createGraph(int V) {
Graph* graph = (Graph*)malloc(sizeof(Graph));
graph->V = V;
graph->E = 0;
for (int i = 0; i < V; i++) {
graph->adjList[i = NULL;
}
return graph;
}
void addEdge(Graph* graph, int src, int dest, int weight) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->vertex = dest;
newNode->weight = weight;
newNode->next = graph->adjList[src];
graph->adjList[src = newNode;
}
void dijkstra(Graph* graph, int start) {
int distance[MAX];
bool visited[MAX];
for (int i = 0; i < graph->V; i++) {
distance[i = INF;
visited[i = false;
}
distance[start = 0;
for (int count = 0; count < graph->V - 1; count++) {
int minDistance = INF;
int minIndex;
for (int v = 0; v < graph->V; v++) {
if (visited[v == false && distance[v <= minDistance) {
minDistance = distance[v];
minIndex = v;
}
}
visited[minIndex = true;
Node* temp = graph->adjList[minIndex];
while (temp != NULL) {
int v = temp->vertex;
int weight = temp->weight;
if (visited[v == false && distance[minIndex != INF && distance[minIndex + weight < distance[v]) {
distance[v = distance[minIndex + weight;
}
temp = temp->next;
}
}
printf("Vertex\tDistance from Start\n");
for (int i = 0; i < graph->V; i++) {
printf("%d\t%d\n", i, distance[i]);
}
}
int main() {
int V = 5; // 顶点数
Graph* graph = createGraph(V);
addEdge(graph, 0, 1, 10);
addEdge(graph, 0, 4, 5);
addEdge(graph, 1, 2, 1);
addEdge(graph, 1, 4, 2);
addEdge(graph, 2, 3, 4);
addEdge(graph, 3, 2, 6);
addEdge(graph, 3, 0, 7);
addEdge(graph, 4, 1, 3);
addEdge(graph, 4, 2, 9);
addEdge(graph, 4, 3, 2);
int start = 0; // 起始顶点
dijkstra(graph, start);
return 0;
}
#### 引用[.reference_title]
- *1* *2* [Dijkstra算法&&邻接表数组](https://blog.csdn.net/qq_30182475/article/details/93346088)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
- *3* [Dijkstra算法(一)之 C语言详解](https://blog.csdn.net/m0_37156901/article/details/103623342)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
相关推荐
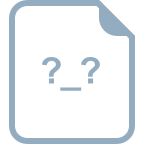
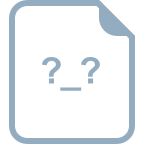














