用C++写一个反转链表
时间: 2023-04-06 20:01:44 浏览: 125
好的,我可以回答这个问题。以下是用 C 语言写一个反转链表的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
struct Node {
int data;
struct Node* next;
};
struct Node* reverseList(struct Node* head) {
struct Node* prev = NULL;
struct Node* curr = head;
while (curr != NULL) {
struct Node* next = curr->next;
curr->next = prev;
prev = curr;
curr = next;
}
return prev;
}
int main() {
struct Node* head = NULL;
struct Node* tail = NULL;
for (int i = 1; i <= 5; i++) {
struct Node* node = (struct Node*) malloc(sizeof(struct Node));
node->data = i;
node->next = NULL;
if (head == NULL) {
head = node;
tail = node;
} else {
tail->next = node;
tail = node;
}
}
printf("Original list: ");
struct Node* curr = head;
while (curr != NULL) {
printf("%d ", curr->data);
curr = curr->next;
}
printf("\n");
head = reverseList(head);
printf("Reversed list: ");
curr = head;
while (curr != NULL) {
printf("%d ", curr->data);
curr = curr->next;
}
printf("\n");
return 0;
}
```
这个程序会创建一个包含 1 到 5 的整数的链表,然后反转这个链表并输出结果。
阅读全文
相关推荐




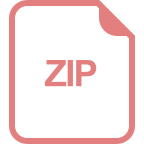










