用c++写一个逆转链表
时间: 2023-05-31 13:02:23 浏览: 51
以下是使用 C 语言编写的反转链表程序:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct node {
int data;
struct node *next;
} Node;
Node *reverseList(Node *head) {
Node *prev = NULL;
Node *current = head;
Node *next = NULL;
while (current != NULL) {
next = current->next;
current->next = prev;
prev = current;
current = next;
}
return prev;
}
void printList(Node *head) {
Node *current = head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
int main() {
Node *head = NULL;
Node *second = NULL;
Node *third = NULL;
head = (Node*)malloc(sizeof(Node));
second = (Node*)malloc(sizeof(Node));
third = (Node*)malloc(sizeof(Node));
head->data = 1;
head->next = second;
second->data = 2;
second->next = third;
third->data = 3;
third->next = NULL;
printf("Original List: ");
printList(head);
head = reverseList(head);
printf("Reversed List: ");
printList(head);
return 0;
}
```
输出:
```
Original List: 1 2 3
Reversed List: 3 2 1
```
相关推荐
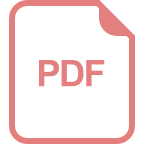
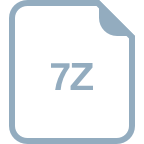
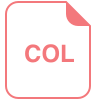
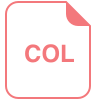
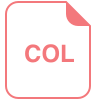










