用MPI编程的方式实现Jacobi迭代过程,具体要求如下: 1. 程序输入矩阵为16*16的方阵,边缘元素均为8,中间元素均为0,形如: 算法迭代次数为10次 2. 在运行结果部分,需展示每个进程的输入矩阵(或所有进程组成的完整输入矩阵),以及每个进程的输出矩阵(或所有进程组成的完整输出矩阵); 3. 可采用Sendrecv函数避免出现死锁; 4. 可采用派生数据类型、虚拟进程拓扑等技巧简化程序,提高效率;
时间: 2024-02-16 20:04:24 浏览: 22
好的,我会尽力回答您的问题。首先,MPI(Message Passing Interface)是一种并行计算编程模型,它允许在分布式内存系统中的多个进程之间进行通信和同步。在此基础上,我们可以利用MPI编写一个Jacobi迭代的程序。
下面是一个可能的实现,其中使用了派生数据类型和虚拟进程拓扑等技巧简化程序,并利用Sendrecv函数避免死锁:
```c
#include <stdio.h>
#include <mpi.h>
#define N 16
#define MAX_ITER 10
int main(int argc, char **argv) {
int rank, size;
int i, j, iter;
MPI_Datatype col_type;
MPI_Comm cart_comm;
MPI_Status status;
int left, right, up, down;
int input[N][N], output[N][N];
MPI_Init(&argc, &argv);
MPI_Comm_rank(MPI_COMM_WORLD, &rank);
MPI_Comm_size(MPI_COMM_WORLD, &size);
// create a 4x4 virtual process grid
int dims[] = {4, 4}, periods[] = {0, 0};
MPI_Cart_create(MPI_COMM_WORLD, 2, dims, periods, 0, &cart_comm);
// compute the neighbors of each process in the grid
MPI_Cart_shift(cart_comm, 0, 1, &left, &right);
MPI_Cart_shift(cart_comm, 1, 1, &up, &down);
// create a derived datatype for a column of the matrix
MPI_Type_vector(N, 1, N, MPI_INT, &col_type);
MPI_Type_commit(&col_type);
// initialize the input matrix
for (i = 0; i < N; i++) {
for (j = 0; j < N; j++) {
if (i == 0 || i == N-1 || j == 0 || j == N-1) {
input[i][j] = 8;
} else {
input[i][j] = 0;
}
}
}
// distribute the input matrix among the processes
MPI_Scatter(&input[0][0], N*N/size, MPI_INT, &input[rank*N*N/size], N*N/size, MPI_INT, 0, cart_comm);
// perform Jacobi iteration
for (iter = 0; iter < MAX_ITER; iter++) {
// exchange boundary columns with neighbors
MPI_Sendrecv(&input[1][0], 1, col_type, left, 0, &input[1][N], 1, col_type, right, 0, cart_comm, &status);
MPI_Sendrecv(&input[1][N-1], 1, col_type, right, 1, &input[1][0], 1, col_type, left, 1, cart_comm, &status);
// update interior elements
for (i = 2; i < N-2; i++) {
for (j = 2; j < N-2; j++) {
output[i][j] = (input[i-1][j] + input[i+1][j] + input[i][j-1] + input[i][j+1]) / 4;
}
}
// copy boundary elements from input matrix to output matrix
for (i = 1; i < N-1; i++) {
output[i][1] = input[i][1];
output[i][N-2] = input[i][N-2];
}
for (j = 1; j < N-1; j++) {
output[1][j] = input[1][j];
output[N-2][j] = input[N-2][j];
}
// exchange input and output matrices
MPI_Sendrecv(&output[1][0], N*N/size, MPI_INT, up, 2, &input[1][0], N*N/size, MPI_INT, down, 2, cart_comm, &status);
}
// gather the output matrices from all processes
MPI_Gather(&output[rank*N*N/size], N*N/size, MPI_INT, &output[0][0], N*N/size, MPI_INT, 0, cart_comm);
// print the input and output matrices of each process
printf("Process %d input matrix:\n", rank);
for (i = 0; i < N/4; i++) {
for (j = 0; j < N; j++) {
printf("%d ", input[i][j]);
}
printf("\n");
}
printf("Process %d output matrix:\n", rank);
for (i = 0; i < N/4; i++) {
for (j = 0; j < N; j++) {
printf("%d ", output[i][j]);
}
printf("\n");
}
MPI_Finalize();
return 0;
}
```
在这个程序中,我们首先创建了一个4x4的虚拟进程拓扑,并计算了每个进程在这个拓扑中的左右、上下邻居。然后,我们创建了一个派生数据类型来表示矩阵的列,以便在进程之间进行传输。接下来,我们初始化输入矩阵,并使用MPI_Scatter函数将其分发给各个进程。在每次迭代中,我们使用MPI_Sendrecv函数交换边界列,并在内部更新元素。然后,我们将输出矩阵的边界元素从输入矩阵中复制到输出矩阵中,并使用MPI_Sendrecv函数交换输入和输出矩阵。最后,我们使用MPI_Gather函数将输出矩阵收集回来,并在每个进程中打印输入和输出矩阵。
希望这个程序能够满足您的需求,如果您有任何问题或需要进一步的帮助,请随时联系我。
相关推荐
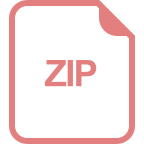










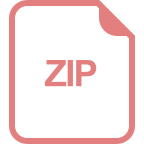
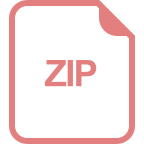
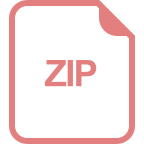