用python写一个网格密度聚类算法
时间: 2024-05-09 09:18:11 浏览: 14
Sure! 网格密度聚类,也被称为DBSCAN算法,是一种聚类算法,通过将样本空间划分为一个个网格,对每个网格中的样本进行密度聚类,来实现分类的目的。在Python中实现网格密度聚类算法,可以使用scikit-learn库中的DBSCAN类。下面是基本的示例代码:
```python
from sklearn.cluster import DBSCAN
import numpy as np
# 加载数据
X = np.array([[1, 2], [2, 2], [2, 3], [8, 7], [8, 8], [25, 80]])
# 创建DBSCAN对象
dbscan = DBSCAN(eps=3, min_samples=2)
# 训练模型
dbscan.fit(X)
# 打印聚类结果
labels = dbscan.labels_
print(labels)
```
这里我们使用了一个简单的二维数据,使用DBSCAN进行聚类。其中,eps参数表示聚类的半径,min_samples表示最小样本数,labels表示每个样本所属的类别。
相关问题
用python写一个密度聚类的算法
好的,可以为您讲解如何用Python写一个密度聚类的算法。密度聚类是一种无良心分类算法,它可以将数据点聚集成密集的区域。在Python中,可以使用scikit-learn库中的DBSCAN类来实现密度聚类算法。具体实现细节可以参考这个文档:https://scikit-learn.org/stable/modules/generated/sklearn.cluster.DBSCAN.html
用python写一个简单的聚类算法
好的,我可以给你一个简单的聚类算法示例,基于K-means算法实现。以下是示例代码:
```python
import numpy as np
from sklearn.cluster import KMeans
# 生成随机数据
X = np.random.rand(100, 2)
# 聚类
kmeans = KMeans(n_clusters=3)
kmeans.fit(X)
# 打印聚类结果
print(kmeans.labels_)
```
上述代码中,我们首先生成了一个100行2列的随机数据矩阵X。然后,我们使用sklearn库中的KMeans类来进行聚类操作,设置聚类数量为3。最后,我们打印出聚类结果,其中每个样本点被标记为0、1或2中的一个。
当然,这只是一个简单的示例,实际应用中还需要进行数据预处理、参数调整等操作。如果你想了解更多聚类算法的细节,可以参考sklearn库中的其他聚类算法,如DBSCAN、MeanShift等。
相关推荐
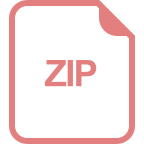
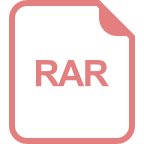












