import pytesseract from PIL import Image # 打开图片 img = Image.open('image.jpg') # 转换成灰度图像 img = img.convert('L') # 识别文字 text = pytesseract.image_to_string(img) # 打印识别结果 print(text)优化
时间: 2024-01-26 09:05:21 浏览: 70
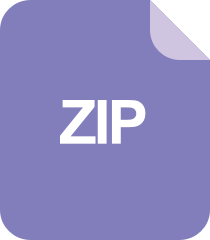
使用 Python 和 pytesseract 进行图片文字识别
在使用 pytesseract 进行图像识别时,可以进行一些优化来提高识别准确率:
1. 对图片进行预处理,例如去除噪点、调整亮度、对比度等等,以便文字更清晰可见;
2. 指定识别语言,例如英文、中文等等;
3. 对于特定的字体和排版,可以训练模型以提高识别准确率;
4. 选择适当的识别模式,例如只识别数字、只识别大写字母等等。
以下是一个优化过的代码示例:
```python
import pytesseract
from PIL import Image, ImageEnhance, ImageFilter
# 打开图片
img = Image.open('image.jpg')
# 预处理图片,去除噪点,提高对比度和亮度
img = img.convert('L')
img = ImageEnhance.Contrast(img).enhance(2)
img = ImageEnhance.Brightness(img).enhance(1.5)
img = img.filter(ImageFilter.MedianFilter())
# 选择识别语言为中文
text = pytesseract.image_to_string(img, lang='chi_sim')
# 打印识别结果
print(text)
```
此外,还可以通过调整 pytesseract 的参数来进一步优化识别效果,例如调整识别阈值、指定识别区域等等。具体可参考 pytesseract 的官方文档。
阅读全文
相关推荐
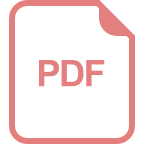















