在python中如何将一个列表变成一个链表
时间: 2023-05-27 07:07:07 浏览: 86
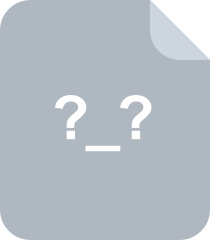
python实现重排链表.md
在 Python 中,可以使用链表的节点类来创建链表。具体步骤如下:
1. 创建节点类,包含一个值属性和一个指向下一个节点的指针属性。
2. 遍历列表,依次创建节点,并将前一个节点的指针指向当前节点。
3. 返回链表的头节点。
下面是代码示例:
```python
class Node:
def __init__(self, value):
self.value = value
self.next = None
def list_to_linkedlist(lst):
# 创建头节点
head = Node(lst[0])
# 遍历列表,创建节点
cur_node = head
for i in range(1, len(lst)):
new_node = Node(lst[i])
cur_node.next = new_node
cur_node = new_node
return head
```
使用示例:
```python
lst = [1, 2, 3, 4, 5]
head = list_to_linkedlist(lst)
# 遍历链表
cur_node = head
while cur_node:
print(cur_node.value)
cur_node = cur_node.next
```
输出:
```
1
2
3
4
5
```
阅读全文
相关推荐
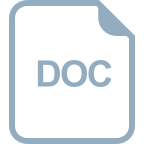
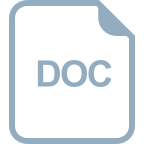
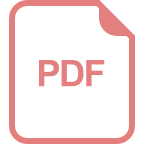
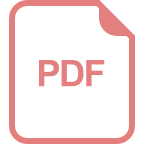
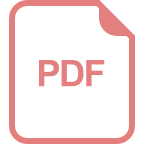
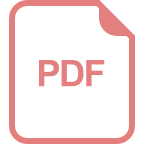











