用C++使用opencv4.7.0生成一个能框出图片中人体轮廓的程序
时间: 2023-05-25 09:03:18 浏览: 210
首先需要安装OpenCV 4.7.0版本及以上,并包含相关的头文件和库文件。
以下是一个示例代码,实现了使用背景减除法和形态学处理,检测出图片中的人体轮廓,并用矩形框标注出来:
```
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace cv;
using namespace std;
int main(int argc, char** argv)
{
// Read image
Mat img = imread("test.jpg");
if (img.empty())
{
cout << "Could not read the image" << endl;
return -1;
}
// Create a background model
Ptr<BackgroundSubtractor> pBackSub;
pBackSub = createBackgroundSubtractorKNN();
// Apply background subtraction
Mat fgMask;
pBackSub->apply(img, fgMask, 0.01); // Set learning rate
// Apply morphological opening to remove noise
Mat kernel = getStructuringElement(MORPH_ELLIPSE, Size(5, 5));
morphologyEx(fgMask, fgMask, MORPH_OPEN, kernel);
// Find contours
vector<vector<Point>> contours;
findContours(fgMask, contours, RETR_EXTERNAL, CHAIN_APPROX_SIMPLE);
// Draw bounding boxes around contours
for (int i = 0; i < contours.size(); i++)
{
Rect rect = boundingRect(contours[i]);
rectangle(img, rect, Scalar(0, 255, 0), 2);
}
// Display result
imshow("Result", img);
waitKey(0);
return 0;
}
```
该代码首先使用KNN背景减除器在输入图像上应用背景消除。然后,通过形态学开运算来移除噪声。接着,通过对象轮廓检测函数 `findContours()` 找到输入图像中的轮廓列表。最后,将每个轮廓放在矩形框中将其在输入图像上显示出来。
需要注意的是,该代码只能检测到人体的轮廓,如果有其他物体也会被检测出来。此外,该代码使用的背景减除算法是KNN背景减除器,而可能还有其他更好的算法可以使用。您可以根据需求和实际情况选择和修改代码。
阅读全文
相关推荐
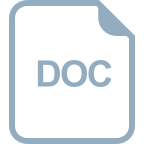
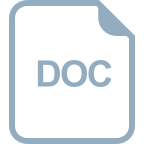
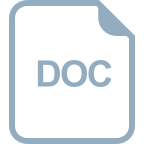


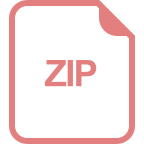
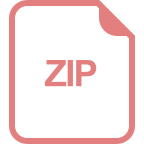
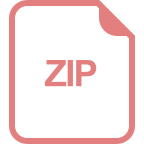
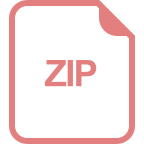
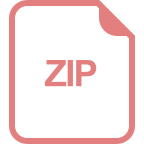
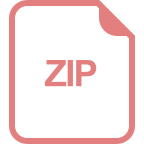
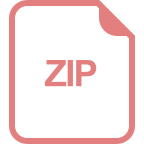
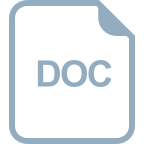
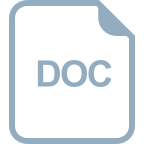
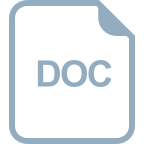
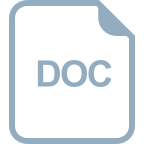

