python中怎么将DataFrame中的数据转换为float
时间: 2024-04-30 12:22:47 浏览: 21
可以使用DataFrame的astype()方法将数据转换为float类型:
``` python
import pandas as pd
# 创建DataFrame
df = pd.DataFrame({'A': ['1.1', '2.2', '3.3'], 'B': ['4.4', '5.5', '6.6']})
# 将数据转换为float类型
df = df.astype(float)
print(df)
```
输出:
```
A B
0 1.1 4.4
1 2.2 5.5
2 3.3 6.6
```
在astype()方法中指定float即可将数据转换为float类型。如果数据中有非数字字符,则会抛出ValueError异常。
相关问题
python将Dataframe转换为浮点数的方法
如果要将整个 DataFrame 中的数据都转换为浮点数,可以使用 DataFrame 的`astype()`方法。例如:
```python
import pandas as pd
# 创建一个包含整数的 DataFrame
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
# 将整数转换为浮点数
df = df.astype(float)
# 输出结果
print(df)
```
输出结果为:
```
A B
0 1.0 4.0
1 2.0 5.0
2 3.0 6.0
```
如果只需要将某一列或几列转换为浮点数,可以使用`astype()`方法的字典形式。例如:
```python
import pandas as pd
# 创建一个包含整数和字符串的 DataFrame
df = pd.DataFrame({'A': [1, 2, 3], 'B': ['4.0', '5.0', '6.0']})
# 将 B 列转换为浮点数
df = df.astype({'B': float})
# 输出结果
print(df)
```
输出结果为:
```
A B
0 1 4.0
1 2 5.0
2 3 6.0
```
需要注意的是,如果字符串不能被转换为浮点数,则会抛出`ValueError`异常。
DataFrame中的某列转换为float类型
可以使用astype()函数将DataFrame中的某列转换为float类型,例如:
```python
import pandas as pd
# 创建一个DataFrame
df = pd.DataFrame({'A': ['1.2', '3.4', '5.6'],
'B': ['7.8', '9.0', '11.2']})
# 将列A转换为float类型
df['A'] = df['A'].astype(float)
# 打印转换后的结果
print(df.dtypes)
```
输出结果为:
```
A float64
B object
dtype: object
```
可以看到,列A已经被成功转换为float类型。
相关推荐
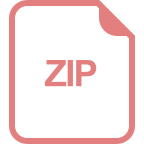
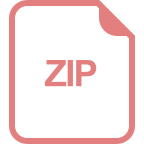
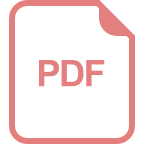
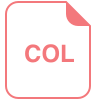
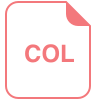
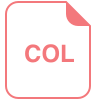









