用java编写程序实现有几何形状边数为n及可计算面积area的 `Shape` 类,其类中类 `Triangle` 类及 `Rectangle` 类实现几何形状三角形和矩形面积area计算,利用前三个形状类实现柱体 `Pillar` 类的体积计算,并在 `PillarTest` 类中实现对某一柱体的体积计算。
时间: 2023-08-12 15:20:03 浏览: 114
好的,这是一个关于Java编程的问题。下面是相应的代码实现:
```java
//Shape.java
public abstract class Shape {
protected int n; // 边数
public abstract double getArea(); // 计算面积
}
//Triangle.java
public class Triangle extends Shape {
private double a, b, c; // 三角形三边长
public Triangle(double a, double b, double c){
this.a = a;
this.b = b;
this.c = c;
this.n = 3;
}
public double getArea(){
double p = (a + b + c) / 2;
return Math.sqrt(p * (p - a) * (p - b) * (p - c));
}
}
//Rectangle.java
public class Rectangle extends Shape {
private double width, height; // 矩形宽和高
public Rectangle(double width, double height){
this.width = width;
this.height = height;
this.n = 4;
}
public double getArea(){
return width * height;
}
}
//Pillar.java
public class Pillar {
private Shape bottom; // 底面形状
private double height; // 柱体高
public Pillar(Shape bottom, double height){
this.bottom = bottom;
this.height = height;
}
public double getVolume(){
return bottom.getArea() * height;
}
}
//PillarTest.java
public class PillarTest {
public static void main(String[] args) {
Shape triangle = new Triangle(3, 4, 5);
Shape rectangle = new Rectangle(2, 5);
Pillar pillar = new Pillar(triangle, 10);
System.out.println(pillar.getVolume()); // 输出50
pillar = new Pillar(rectangle, 10);
System.out.println(pillar.getVolume()); // 输出100
}
}
```
在这个代码实现中,`Shape` 是一个抽象类,表示几何形状,包含 `n` 表示边数和 `getArea()` 方法用于计算面积。`Triangle` 和 `Rectangle` 继承自 `Shape` 类,分别表示三角形和矩形,实现了 `getArea()` 方法用于计算面积。`Pillar` 类表示柱体,包含底面形状和高,实现了 `getVolume()` 方法用于计算体积。`PillarTest` 类则用于测试柱体的体积计算。
相关推荐














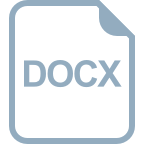
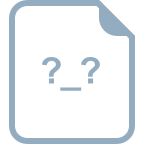
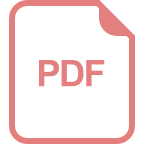
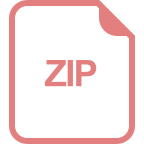