生成一段将矩阵保存为png的pyhon代码
时间: 2024-05-04 16:15:47 浏览: 88
当你生成矩阵之后,可以使用以下代码将其保存为png图片:
```
import numpy as np
from PIL import Image
# 生成矩阵
matrix = np.random.rand(10, 10)
# 将矩阵转换为图像对象
matrix_image = Image.fromarray((matrix * 255).astype(np.uint8))
# 保存为png格式图片
matrix_image.save('matrix.png')
```
希望这个代码对你有帮助!
相关问题
生成一段调用gdal将矩阵保存为png的pyhon代码
以下是一段将矩阵保存为PNG格式的Python代码,其中使用了GDAL库:
```python
import gdal
import numpy as np
# 要保存的矩阵
matrix = np.array([[1, 2], [3, 4]])
# 定义输出PNG文件名和路径
output_file = "/path/to/output.png"
# 定义输出驱动,这里选择PNG格式
driver = gdal.GetDriverByName("PNG")
# 计算矩阵的行数和列数
rows, cols = matrix.shape
# 创建输出数据集,指定行数、列数和波段数(这里为1)
dataset = driver.Create(output_file, cols, rows, 1, gdal.GDT_Byte)
# 获取输出数据集的第一个波段
band = dataset.GetRasterBand(1)
# 将矩阵写入输出数据集的第一个波段中
band.WriteArray(matrix)
# 将数据集写入磁盘中
dataset.FlushCache()
```
希望对你有所帮助!
生成一段python代码,输入为根据立体匹配得到的视差图与相机标定参数,进行双目三维重建
以下是一个简单的 Python 代码示例,用于根据立体匹配得到的视差图和相机标定参数进行双目三维重建:
```python
import cv2
import numpy as np
# 读取左右视图图像和视差图
left_img = cv2.imread('left.jpg', cv2.IMREAD_GRAYSCALE)
right_img = cv2.imread('right.jpg', cv2.IMREAD_GRAYSCALE)
disparity = cv2.imread('disparity.png', cv2.IMREAD_GRAYSCALE)
# 读取相机标定参数
focal_length = 1000 # 相机焦距
baseline = 0.1 # 相机基线长度
# 计算深度图
depth = np.zeros_like(disparity).astype(np.float32)
mask = disparity > 0
depth[mask] = focal_length * baseline / disparity[mask]
# 计算点云
points = cv2.reprojectImageTo3D(disparity, Q)
points = points.reshape(-1, 3)
points = points[mask]
# 显示点云
from mpl_toolkits.mplot3d import Axes3D
import matplotlib.pyplot as plt
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ax.scatter(points[:, 0], points[:, 1], points[:, 2], s=0.1)
ax.set_xlabel('X')
ax.set_ylabel('Y')
ax.set_zlabel('Z')
plt.show()
```
其中,Q 矩阵是由相机标定参数计算得到的,可以使用 OpenCV 的 `cv2.stereoRectify()` 函数进行计算。通过计算深度图和点云,可以将双目图像转换为三维空间中的点云,从而实现三维重建。
阅读全文
相关推荐
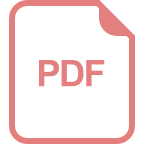
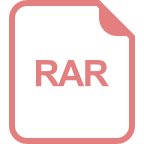
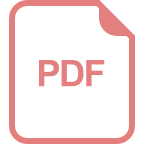
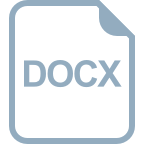
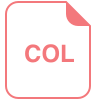
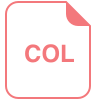
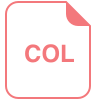
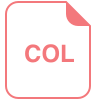
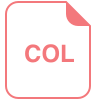
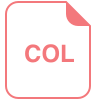
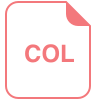





