esp32 arduino mqtt
时间: 2023-09-11 08:04:52 浏览: 108
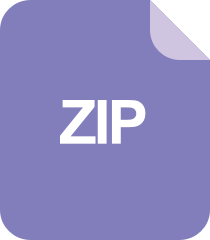
ARDUINO-ESP32
ESP32 Arduino MQTT is a way to connect an ESP32 microcontroller to an MQTT broker using the Arduino programming language. MQTT stands for Message Queuing Telemetry Transport, which is a lightweight messaging protocol designed for IoT devices. This protocol allows devices to publish and subscribe to topics, enabling communication between them.
To use ESP32 Arduino MQTT, you need to first set up your ESP32 microcontroller with the Arduino IDE. Once you have done that, you can install the MQTT library for Arduino and start coding. Here are the basic steps:
1. Install the PubSubClient library in the Arduino IDE.
2. Connect your ESP32 to a Wi-Fi network using the Wi-Fi library.
3. Connect to an MQTT broker using the MQTT library and the PubSubClient library.
4. Publish messages to a topic using the MQTT library and the PubSubClient library.
5. Subscribe to topics and receive messages using the MQTT library and the PubSubClient library.
Here is an example code for setting up an ESP32 Arduino MQTT connection:
```
#include <WiFi.h>
#include <PubSubClient.h>
// Wi-Fi credentials
const char* ssid = "your_SSID";
const char* password = "your_PASSWORD";
// MQTT broker details
const char* mqtt_server = "your_MQTT_broker_IP_address";
const int mqtt_port = 1883;
const char* mqtt_username = "your_MQTT_username";
const char* mqtt_password = "your_MQTT_password";
// MQTT client
WiFiClient espClient;
PubSubClient client(espClient);
void setup() {
// Connect to Wi-Fi network
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
}
// Connect to MQTT broker
client.setServer(mqtt_server, mqtt_port);
client.setCallback(callback);
while (!client.connected()) {
if (client.connect("ESP32_client", mqtt_username, mqtt_password)) {
client.subscribe("test/topic");
} else {
delay(1000);
}
}
}
void loop() {
// Publish message to MQTT broker
client.publish("test/topic", "Hello, world!");
// Check for incoming messages
client.loop();
}
void callback(char* topic, byte* payload, unsigned int length) {
// Handle incoming messages
Serial.print("Message received on topic: ");
Serial.println(topic);
Serial.print("Message: ");
for (int i = 0; i < length; i++) {
Serial.print((char)payload[i]);
}
Serial.println();
}
```
This code connects to a Wi-Fi network and an MQTT broker, subscribes to a topic, publishes a message to that topic, and receives incoming messages. You can modify the code to fit your specific use case.
阅读全文
相关推荐
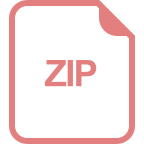




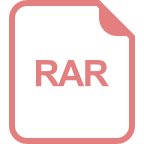
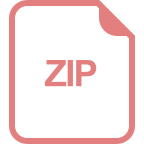
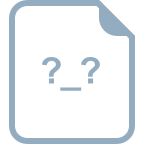
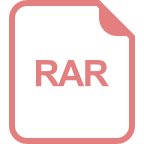
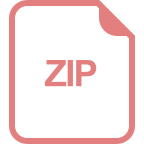





