写一个二叉排序树c语言算法实现
时间: 2023-11-22 08:59:15 浏览: 82
好的,下面是实现二叉排序树的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 二叉排序树节点结构体
typedef struct node {
int data;
struct node* lchild;
struct node* rchild;
}Node;
// 创建一个节点
Node* createNode(int val) {
Node* new_node = (Node*)malloc(sizeof(Node));
new_node->data = val;
new_node->lchild = NULL;
new_node->rchild = NULL;
return new_node;
}
// 插入节点
Node* insert(Node* root, int val) {
if (root == NULL) {
root = createNode(val);
return root;
}
if (val == root->data) {
return root;
} else if (val < root->data) {
root->lchild = insert(root->lchild, val);
} else {
root->rchild = insert(root->rchild, val);
}
return root;
}
// 中序遍历
void inorderTraversal(Node* root) {
if (root == NULL) return;
inorderTraversal(root->lchild);
printf("%d ", root->data);
inorderTraversal(root->rchild);
}
int main() {
Node* root = NULL;
// 插入节点
root = insert(root, 8);
insert(root, 3);
insert(root, 10);
insert(root, 1);
insert(root, 6);
insert(root, 14);
insert(root, 4);
insert(root, 7);
insert(root, 13);
// 中序遍历
inorderTraversal(root);
return 0;
}
```
希望对你有所帮助!
阅读全文
相关推荐
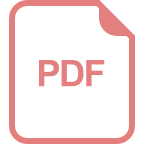
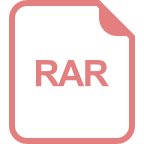
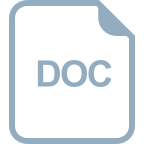
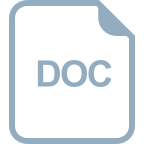
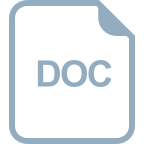









