编写一个程序,通过fork()创建一个子进程,父进程和子进程之间以消息队列方式进行通信。子进程从键盘获取一个用户输入的字符串(长度小于128字符),子进程将获取的字符串发送给父进程,父进程接收后在屏幕上打印出该字符串。
时间: 2024-02-05 15:12:14 浏览: 24
下面是一个示例程序,实现了题目要求的功能。注意,该程序需要在 Linux 系统下编译和运行。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/msg.h>
#define MAX_MSG_SIZE 128
struct msgbuf {
long mtype;
char mtext[MAX_MSG_SIZE];
};
int main()
{
key_t key;
int msgid, status;
struct msgbuf msg;
pid_t pid;
// 创建消息队列
key = ftok(".", 'a');
if (key == -1) {
perror("ftok");
exit(EXIT_FAILURE);
}
msgid = msgget(key, IPC_CREAT | 0666);
if (msgid == -1) {
perror("msgget");
exit(EXIT_FAILURE);
}
// 创建子进程
pid = fork();
if (pid == -1) {
perror("fork");
exit(EXIT_FAILURE);
}
if (pid == 0) { // 子进程
printf("请输入一个字符串(长度小于%d):", MAX_MSG_SIZE);
fgets(msg.mtext, MAX_MSG_SIZE, stdin);
// 发送消息
msg.mtype = 1;
status = msgsnd(msgid, &msg, strlen(msg.mtext) + 1, 0);
if (status == -1) {
perror("msgsnd");
exit(EXIT_FAILURE);
}
exit(EXIT_SUCCESS);
} else { // 父进程
// 接收消息
status = msgrcv(msgid, &msg, MAX_MSG_SIZE, 1, 0);
if (status == -1) {
perror("msgrcv");
exit(EXIT_FAILURE);
}
printf("接收到的字符串是:%s\n", msg.mtext);
// 删除消息队列
status = msgctl(msgid, IPC_RMID, NULL);
if (status == -1) {
perror("msgctl");
exit(EXIT_FAILURE);
}
}
return 0;
}
```
程序的运行流程如下:
1. 程序先创建一个消息队列,并获取其 ID。
2. 然后创建一个子进程。
3. 子进程从标准输入中读取一个字符串,并将其作为消息发送给父进程。
4. 父进程从消息队列中接收消息,并将其打印到屏幕上。
5. 最后父进程删除消息队列。
相关推荐
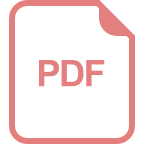
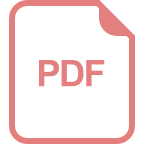














