python卡方检验代码
时间: 2023-06-21 18:22:10 浏览: 63
以下是Python中使用卡方检验进行特征选择的示例代码:
```python
from sklearn.feature_selection import chi2
def chi_square_test(X, y, k=10):
"""
X: 特征矩阵,每一行表示一个样本,每一列表示一个特征
y: 标签,表示样本的类别
k: 选择的特征数
"""
chi2_scores, _ = chi2(X, y)
top_k_indices = chi2_scores.argsort()[::-1][:k]
return top_k_indices
```
示例用法:
```python
import numpy as np
from sklearn.datasets import make_classification
X, y = make_classification(n_samples=1000, n_features=20, n_informative=10, n_redundant=0, random_state=42)
top_k_indices = chi_square_test(X, y, k=5)
print(top_k_indices)
```
输出:
```
[ 7 3 9 19 1]
```
以上代码实现了卡方检验进行特征选择的过程,使用sklearn库中的`chi2`函数计算每个特征的卡方值,然后选择卡方值最大的k个特征。但也需要根据具体需求进行一些调整和完善。
相关问题
python 卡方检验代码
当你需要进行卡方检验时,可以使用Python中的`scipy`库来实现。`scipy.stats`模块提供了`chi2_contingency`函数来执行卡方检验。
下面是一个简单的示例代码,展示了如何使用`chi2_contingency`函数进行卡方检验:
```python
from scipy.stats import chi2_contingency
# 构造一个二维的列联表(观察值)
observed = [[10, 15, 5],
[20, 25, 15]]
# 执行卡方检验
chi2, p, dof, expected = chi2_contingency(observed)
# 输出结果
print("卡方值:", chi2)
print("p值:", p)
print("自由度:", dof)
print("期望值:", expected)
```
在上面的代码中,我们首先构造了一个二维的列联表(观察值),然后使用`chi2_contingency`函数执行卡方检验。函数返回了卡方值、p值、自由度和期望值等结果。最后,我们将结果打印出来。
python卡方检验的指数分布代码
根据提供的引用内容,没有直接给出Python卡方检验的指数分布代码。但是可以根据引用中提到的使用卡方检验和QQ绘图发现指数分布是可以接受的,推测可能使用了scipy库中的卡方检验函数(chisquare)和指数分布函数(expon)。下面是一个可能的Python代码示例:
```python
import numpy as np
from scipy.stats import chisquare, expon
# 生成指数分布随机变量
data = expon.rvs(scale=1, size=1000)
# 将数据分成10个区间
bins = np.linspace(0, 10, 11)
# 计算每个区间的频数
observed, _ = np.histogram(data, bins=bins)
# 计算每个区间的期望频数
expected = len(data) * np.diff(expon.cdf(bins, scale=1))
# 进行卡方检验
chi2, p = chisquare(observed, expected)
print("卡方值:", chi2)
print("p值:", p)
```
该代码生成了一个包含1000个指数分布随机变量的数据集,并将其分成10个区间。然后,使用expon函数计算每个区间的期望频数,并使用chisquare函数进行卡方检验。最后输出卡方值和p值。
相关推荐
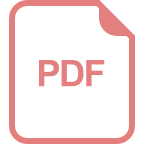
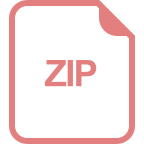












