在C#下使用TensorFlow.NET训练自己的数据集例程
时间: 2023-09-13 22:04:34 浏览: 182
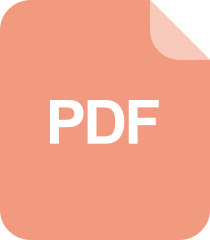
C#使用TensorFlow.NET训练自己的数据集的方法
好的,下面是使用TensorFlow.NET训练自己的数据集的例程:
1. 准备数据集
首先,需要准备自己的数据集。数据集应该包括训练集和测试集两部分,每个部分应该包括输入数据和标签。
2. 安装TensorFlow.NET
可以在官网下载最新版本的TensorFlow.NET,并按照官方文档进行安装。
3. 构建模型
使用TensorFlow.NET构建模型的方法与使用TensorFlow类似。可以使用Keras API或者直接调用TensorFlow.NET的API。
下面是一个使用Keras API构建模型的例子:
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using TensorFlow;
using Keras;
using Keras.Layers;
using Keras.Models;
namespace TensorFlowNET.Examples
{
class Program
{
static void Main(string[] args)
{
var (x_train, y_train) = LoadData("train.csv");
var (x_test, y_test) = LoadData("test.csv");
var input = new Input(new TensorShape(28, 28));
var x = new Reshape(new int[] { 28 * 28 }).Apply(input);
x = new Dense(128, activation: "relu").Apply(x);
x = new Dense(10, activation: "softmax").Apply(x);
var model = new Model(input, x);
model.Compile(optimizer: "adam", loss: "categorical_crossentropy", metrics: new[] { "accuracy" });
model.Fit(x_train, y_train, batch_size: 128, epochs: 5, validation_split: 0.1);
var score = model.Evaluate(x_test, y_test);
Console.WriteLine($"Test loss: {score[0]}");
Console.WriteLine($"Test accuracy: {score[1]}");
}
static (NDArray, NDArray) LoadData(string file)
{
// Load data from file
return (x, y);
}
}
}
```
4. 训练模型
使用准备好的数据集和构建好的模型进行训练。可以使用模型的`Fit`方法进行批量训练。
```csharp
model.Fit(x_train, y_train, batch_size: 128, epochs: 5, validation_split: 0.1);
```
5. 评估模型
在训练完成后,可以使用模型的`Evaluate`方法对模型进行评估。
```csharp
var score = model.Evaluate(x_test, y_test);
Console.WriteLine($"Test loss: {score[0]}");
Console.WriteLine($"Test accuracy: {score[1]}");
```
以上就是使用TensorFlow.NET训练自己的数据集的例程。希望对你有帮助!
阅读全文
相关推荐

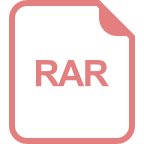
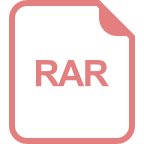
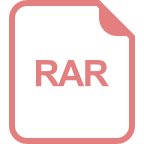
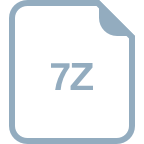
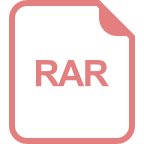
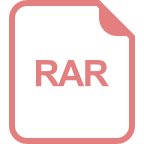
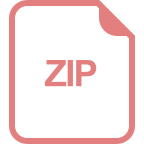
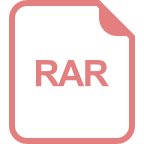
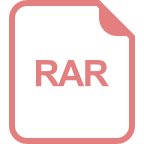
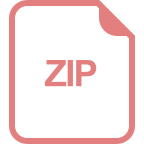
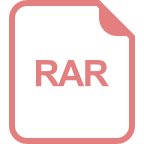
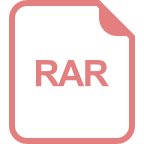