在ros中用c++实现订阅给定的topic是否有数据,但是事先不知道该topic的类型
时间: 2023-06-10 20:07:10 浏览: 183
在ROS中,可以使用`rostopic`命令行工具来查询给定topic的类型。但是如果不知道topic的类型,可以使用C API中的`ros::master::getTopicTypes`函数来获取所有已知topic的类型。以下是一个示例代码,可以查询给定的topic是否存在,如果存在,就输出该topic的类型:
```c
#include <ros/ros.h>
#include <ros/master.h>
int main(int argc, char** argv)
{
// Initialize ROS
ros::init(argc, argv, "topic_type_query");
if(argc != 2)
{
ROS_ERROR("Usage: topic_type_query <topic_name>");
return 1;
}
// Get list of all topics
ros::V_string topics;
ros::master::getTopics(topics);
// Look for specified topic
std::string topic_name = argv[1];
std::string topic_type = "";
for(auto it = topics.begin(); it != topics.end(); ++it)
{
std::string topic = *it;
if(topic.find(topic_name) != std::string::npos)
{
// Get type of topic
ros::master::V_TopicInfo topic_info;
ros::master::getTopicTypes(topic, topic_info);
if(topic_info.size() > 0)
{
topic_type = topic_info[0].datatype;
break;
}
}
}
if(topic_type.empty())
{
ROS_INFO("Topic '%s' not found.", topic_name.c_str());
}
else
{
ROS_INFO("Topic '%s' has type '%s'.", topic_name.c_str(), topic_type.c_str());
}
return 0;
}
```
这个例子中,`ros::master::getTopics`函数获取了所有已知的topics,并使用`std::string::find`函数查找符合给定名称的topic。一旦找到了该topic,就可以使用`ros::master::getTopicTypes`函数来获取该topic的类型信息。如果找不到该topic,就输出一条消息,否则输出该topic的类型信息。
阅读全文
相关推荐
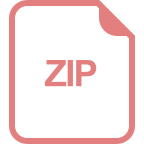
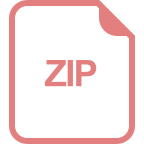
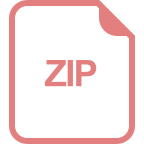

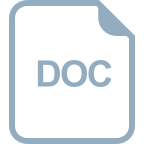


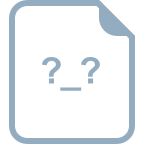
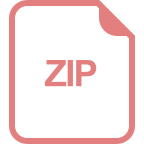
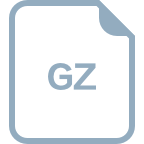
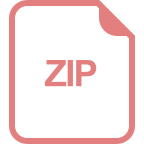
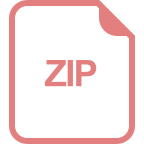
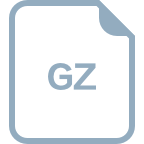
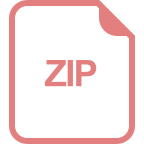
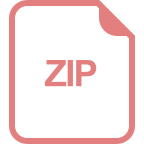
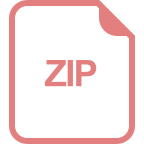
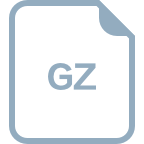
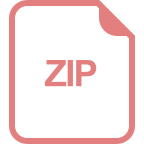
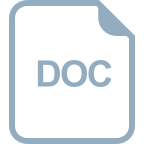