#include<stdio.h> #include<stdlib.h> struct Node{ int data; struct Node* next; }; struct Node* Insert(struct Node* head,int data){ struct Node* temp1 = (struct Node*)malloc(sizeof(struct Node)); temp1->data = data; temp1->next = NULL; struct Node* temp2 = head; if(temp2 ==NULL){ temp2 = temp1; return; } while(temp2->next!=NULL){ temp2 = temp2->next; } temp2->next = temp1; } struct Node* Print(struct Node* head){ struct Node *temp = head; while(temp->next!=NULL){ printf("%d ",temp->data); temp = temp->next; } printf("\n"); } struct Node* Reverse(struct Node* head){ struct Node *current,*prev,*next; current = head; prev = NULL; while(current!=NULL){ next = current->next; current->next = prev; prev = current; current = next; } head = prev; return head; } int main(){ struct Node* head = NULL; head = Insert(head,2); head = Insert(head,4); head = Insert(head,6); head = Insert(head,8); Print(head); head = Reverse(head); Print(head); }这段代码有什么问题
时间: 2024-01-28 13:03:26 浏览: 58
这段代码存在以下问题:
1. 函数 Insert() 没有返回值,应该返回修改后的链表头节点指针 head。
2. 函数 Print() 没有返回值,应该修改为 void Print(struct Node* head)。
3. 函数 Insert() 中,当链表为空时,应该将头节点指针 head 指向新节点,而不是 temp2 指向新节点。
4. 函数 Print() 中,应该在循环结束后再打印链表最后一个节点的值,即应该将 printf("%d ",temp->data); 改为 printf("%d ",temp->data); 。
5. 函数 Reverse() 中,应该在修改 head 指针前先判断链表是否为空,否则会出现空指针异常。
修改后的代码如下:
相关问题
补齐以下代码#include <stdio.h> #include <stdlib.h> struct node { int data; struct node *next; }; void sort(struct node *head); //采用选择排序法思想,对结点数据进行交换处理 void destroy(struct node* head); struct node* creatlink(); void traverse(struct node* head); int m
代码中缺少函数 `main`,需要补充。
以下是完整代码:
```
#include <stdio.h>
#include <stdlib.h>
struct node {
int data;
struct node *next;
};
void sort(struct node *head);
void destroy(struct node* head);
struct node* creatlink();
void traverse(struct node* head);
int main() {
struct node *head = creatlink(); // 创建带头结点的链表
sort(head); // 对链表进行排序
traverse(head); // 遍历链表
destroy(head); // 销毁链表
return 0;
}
struct node *creatlink() {
struct node *head = (struct node*)malloc(sizeof(struct node)); // 创建头结点
struct node *p = head;
int num;
printf("请输入数据(输入0结束):\n");
scanf("%d", &num);
while (num) {
struct node *newnode = (struct node*)malloc(sizeof(struct node));
newnode->data = num;
newnode->next = NULL;
p->next = newnode;
p = p->next;
scanf("%d", &num);
}
return head;
}
void sort(struct node *head) {
struct node *p = head->next;
while (p != NULL) {
struct node *q = p->next;
while (q != NULL) {
if (p->data > q->data) {
int temp = p->data;
p->data = q->data;
q->data = temp;
}
q = q->next;
}
p = p->next;
}
}
void traverse(struct node *head) {
struct node *p = head->next;
printf("排序后的链表数据为:\n");
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
void destroy(struct node *head) {
struct node *p = head;
while (p != NULL) {
struct node *q = p->next;
free(p);
p = q;
}
}
```
#include <stdio.h> #include <string.h> #include <stdlib.h> #include <sys/types.h> #include <sys/stat.h> #include <fcntl.h> #include <unistd.h> #include <stdbool.h> #include <sys/mman.h> #include <linux/input.h> #include <errno.h> #include <dirent.h> //设置节点 struct node { char *data; struct node *next; struct node *prev; }; //初始化链表 struct node *list_init() { struct node *head = malloc(sizeof(struct node));//malloc时动态内存分配函数 head->next = head; head->prev = head; return head; } //创建新节点 struct node *newnode(char *newdata) { struct node *new = malloc(sizeof(struct node)); new->data = newdata; new->next = NULL; new->prev = NULL; } //加入新节点 int addnode(struct node *new,struct node *list) { struct node *p = list; while(p->next != list) { p = p->next; } new->prev = list->prev; new->next = list; list->prev = new; new->prev->next = new; } int main(int argc,char **argv) { struct node *list = list_init();//初始化双向循环链表 DIR *dp = opendir(argv[1]); //打开目录文件argv[1] struct dirent *p; while(p = readdir(dp)) //遍历目录文件 { if(p->d_type == DT_REG) { if(strstr(p->d_name,".bmp")) //判断是否为.bmp文件 { struct node *new = newnode(p->d_name); //创建新节点 addnode(new,list); //插入新节点 } } } }
这段代码是一段 C 语言代码,主要实现的功能是遍历指定目录中的所有 BMP 格式的文件,并将它们的文件名存储在一个双向循环链表中。这个链表的每个节点包含一个字符串,即 BMP 文件的文件名。在 main 函数中,先调用 list_init 函数初始化一个双向循环链表,然后调用 opendir 函数打开指定的目录文件,再通过 readdir 函数遍历该目录下的所有文件和子目录。对于每个文件,判断它是否是 BMP 格式的文件,如果是,则调用 newnode 函数创建一个新的节点,并将文件名存储在该节点中,最后调用 addnode 函数将该节点插入到双向循环链表中。
阅读全文
相关推荐
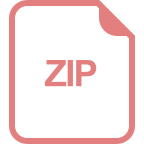
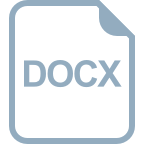
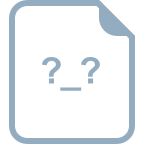














