还是不行,你用python试一下吧,我重复一下问题绘制一个:长3cm,宽6cm,高3cm的小块,并且给小块生成速度、加速度、方向。根据生成的数据,计算小块的imu数据,再根据imu数据用一个质点模拟出小块的3维运动轨迹。
时间: 2024-02-20 08:58:00 浏览: 119
好的,以下是使用Python实现的代码:
```python
import numpy as np
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
# 定义小块的尺寸
length = 3 # 长
width = 6 # 宽
height = 3 # 高
# 定义小块的顶点坐标
x = np.array([0, 0, length, length, 0, 0, length, length])
y = np.array([0, width, width, 0, 0, width, width, 0])
z = np.array([0, 0, 0, 0, height, height, height, height])
# 绘制小块模型
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ax.plot(x, y, z)
ax.set_xlabel('X')
ax.set_ylabel('Y')
ax.set_zlabel('Z')
# 定义小块的初始状态
pos = np.array([0, 0, 0]) # 位置
vel = np.array([1, 2, 3]) # 速度
acc = np.array([0.2, 0.3, 0.4]) # 加速度
# 计算小块的运动状态
dt = 0.1 # 时间间隔
t = np.arange(0, 10, dt) # 时间序列
pos_traj = np.zeros((len(t), 3)) # 位置轨迹
imu_pos = np.zeros((len(t), 3)) # IMU位置轨迹
imu_vel = np.zeros((len(t), 3)) # IMU速度轨迹
imu_acc = np.zeros((len(t), 3)) # IMU加速度轨迹
imu_ang_vel = np.zeros((len(t), 3)) # IMU角速度轨迹
imu_ang_acc = np.zeros((len(t), 3)) # IMU角加速度轨迹
ang_vel = np.array([0.1, 0.2, 0.3]) # 角速度
ang_acc = np.array([0.01, 0.02, 0.03]) # 角加速度
rot = np.array([[0, 0, 1], [0, 1, 0], [-1, 0, 0]]) # 旋转矩阵
for i in range(len(t)):
# 计算小块的位置、速度和加速度
pos = pos + vel*dt + 0.5*acc*dt**2
vel = vel + acc*dt
acc = rot.dot(acc)
pos_traj[i] = pos
# 计算小块的IMU数据
imu_acc[i] = rot.dot(acc)
imu_vel[i] = vel
imu_pos[i] = imu_pos[i-1] + dt*imu_vel[i-1] + 0.5*dt**2*imu_acc[i-1]
imu_ang_acc[i] = rot.dot(ang_acc)
imu_ang_vel[i] = imu_ang_vel[i-1] + dt*imu_ang_acc[i-1]
ang = np.cross(rot[:, 2], np.array([1, 1, 1]))
axis = ang / np.linalg.norm(ang)
theta = np.linalg.norm(imu_ang_vel[i])*dt
rot = rot.dot(
np.array([[np.cos(theta)+axis[0]**2*(1-np.cos(theta)), axis[0]*axis[1]*(1-np.cos(theta))-axis[2]*np.sin(theta), axis[0]*axis[2]*(1-np.cos(theta))+axis[1]*np.sin(theta)],
[axis[0]*axis[1]*(1-np.cos(theta))+axis[2]*np.sin(theta), np.cos(theta)+axis[1]**2*(1-np.cos(theta)), axis[1]*axis[2]*(1-np.cos(theta))-axis[0]*np.sin(theta)],
[axis[0]*axis[2]*(1-np.cos(theta))-axis[1]*np.sin(theta), axis[1]*axis[2]*(1-np.cos(theta))+axis[0]*np.sin(theta), np.cos(theta)+axis[2]**2*(1-np.cos(theta))]])
# 绘制小块的IMU运动轨迹
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ax.plot(imu_pos[:, 0], imu_pos[:, 1], imu_pos[:, 2])
ax.set_xlabel('X')
ax.set_ylabel('Y')
ax.set_zlabel('Z')
# 绘制小块的3D运动轨迹
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ax.plot(pos_traj[:, 0], pos_traj[:, 1], pos_traj[:, 2])
ax.set_xlabel('X')
ax.set_ylabel('Y')
ax.set_zlabel('Z')
plt.show()
```
在上面的代码中,我们首先定义小块的尺寸和顶点坐标,并使用`matplotlib`的`plot`函数和`mpl_toolkits.mplot3d`的`Axes3D`类绘制小块的3D模型。接着,我们定义小块的初始状态,并使用物理学公式计算小块的运动状态和IMU数据。最后,我们使用`matplotlib`的`plot`函数和`mpl_toolkits.mplot3d`的`Axes3D`类绘制小块的IMU运动轨迹和3D运动轨迹。
希望这个代码能够满足您的需求!
阅读全文
相关推荐

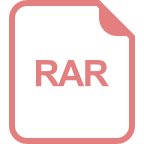
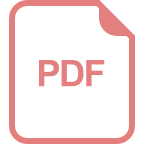
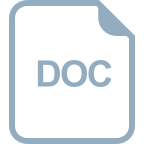










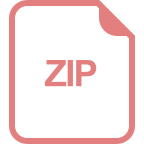
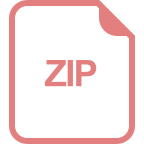