C++ vector string 用逗号相连
时间: 2023-10-22 21:36:57 浏览: 26
可以使用 C++11 中的字符串连接符来实现:
```c++
#include <iostream>
#include <vector>
#include <string>
int main() {
std::vector<std::string> vec = {"hello", "world", "how", "are", "you"};
std::string result;
for (const auto& str : vec) {
if (!result.empty()) {
result += ",";
}
result += str;
}
std::cout << result << std::endl;
return 0;
}
```
这段代码将一个 vector<string> 中的字符串用逗号连接起来并输出。
相关问题
c++ vector<string> 用逗号连接
可以使用以下代码将 `vector<string>` 中的字符串以逗号连接起来:
```c++
#include <iostream>
#include <string>
#include <vector>
std::string join(const std::vector<std::string>& vec, const std::string& delimiter) {
std::string result;
if (!vec.empty()) {
result = vec[0];
for (size_t i = 1; i < vec.size(); ++i) {
result += delimiter + vec[i];
}
}
return result;
}
int main() {
std::vector<std::string> vec = {"hello", "world", "c++", "vector", "string"};
std::string delimiter = ",";
std::cout << join(vec, delimiter) << std::endl;
return 0;
}
```
这个程序定义了一个 `join` 函数,接受一个 `vector<string>` 和一个分隔符作为参数,将其中的字符串以分隔符连接起来,并返回连接后的字符串。程序中的 `main` 函数演示了如何使用 `join` 函数将 `vector<string>` 中的字符串以逗号连接起来。
c++ string逗号分隔转数组
你可以使用 `std::stringstream` 和 `std::getline` 将逗号分隔的字符串转换为数组。下面是一个示例代码:
```c++
#include <iostream>
#include <string>
#include <sstream>
#include <vector>
int main() {
std::string str = "1,2,3,4,5";
std::vector<int> nums;
std::stringstream ss(str);
std::string token;
while (std::getline(ss, token, ',')) {
nums.push_back(std::stoi(token));
}
for (int num : nums) {
std::cout << num << " ";
}
std::cout << std::endl;
return 0;
}
```
输出结果为:
```
1 2 3 4 5
```
在上面的代码中,我们先定义了一个字符串 `str`,它包含了逗号分隔的数字。然后我们定义了一个 `std::vector<int>` 类型的数组 `nums`,用来存储转换后的数字。接着,我们使用 `std::stringstream` 将字符串 `str` 传入,并使用 `std::getline` 函数按照逗号分隔符将字符串分割成多个子串,存储在 `token` 变量中。最后,我们将 `token` 转换为 `int` 类型,并将其添加到数组 `nums` 中。
最后,我们遍历数组 `nums` 并输出每个元素的值。
相关推荐
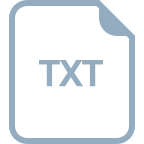
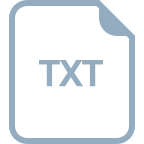
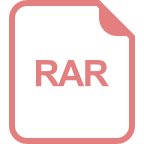












