实现06-bubbles-1.html , 并注释关键技术点
时间: 2023-06-13 19:08:08 浏览: 150
好的,以下是实现06-bubbles-1.html的代码,已经加上了注释:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Bubbles</title>
<style>
body {
margin: 0;
padding: 0;
background-color: #000;
overflow: hidden; /* 隐藏溢出部分 */
cursor: none; /* 隐藏鼠标 */
}
canvas {
display: block; /* 去除默认margin和padding */
position: absolute; /* 绝对定位 */
top: 0;
left: 0;
z-index: -1; /* 在最底层 */
}
</style>
</head>
<body>
<canvas></canvas>
<script>
const canvas = document.querySelector('canvas');
const ctx = canvas.getContext('2d');
// 设置画布的宽度和高度为窗口的宽度和高度
canvas.width = window.innerWidth;
canvas.height = window.innerHeight;
// 定义 Bubble 类
class Bubble {
constructor() {
this.radius = Math.random() * 50 + 10; // 半径在 10 ~ 60 之间随机
this.x = Math.random() * (canvas.width - this.radius * 2) + this.radius; // x 坐标在半径到画布宽度减去半径之间随机
this.y = canvas.height + this.radius; // y 坐标在画布下方
this.speed = Math.random() * 5 + 1; // 速度在 1 ~ 6 之间随机
this.color = `rgba(${Math.random() * 255}, ${Math.random() * 255}, ${Math.random() * 255}, 0.5)`; // 随机颜色
}
update() {
this.y -= this.speed; // 更新 y 坐标
}
draw() {
ctx.beginPath();
ctx.arc(this.x, this.y, this.radius, 0, Math.PI * 2); // 绘制圆形
ctx.fillStyle = this.color;
ctx.fill();
}
}
const bubbles = [];
function createBubble() {
bubbles.push(new Bubble()); // 在 bubbles 数组中增加一个新的 Bubble 实例
setTimeout(createBubble, Math.random() * 1000); // 每隔 0 ~ 1 秒随机生成一个新的 Bubble
}
function animate() {
ctx.clearRect(0, 0, canvas.width, canvas.height); // 清除画布
bubbles.forEach((bubble) => {
bubble.update(); // 更新 Bubble 实例的位置
bubble.draw(); // 绘制 Bubble 实例
});
requestAnimationFrame(animate); // 循环调用 animate 函数
}
createBubble(); // 开始生成 Bubble
animate(); // 开始动画
</script>
</body>
</html>
```
关键技术点注释如下:
- canvas元素:HTML5新增的元素,可以通过JavaScript在其中绘制图形。
- getContext('2d')方法:获取canvas元素的绘图上下文,参数'2d'表示绘制二维图形。
- canvas.width和canvas.height属性:设置canvas元素的宽度和高度,单位为像素。
- Bubble类:定义了气泡的属性和方法。
- Math.random()方法:返回0到1之间的随机数。
- ctx.beginPath()方法:开始绘制路径,路径上的所有绘制操作都会按照顺序被记录下来。
- ctx.arc()方法:绘制圆形路径。
- ctx.fillStyle属性:设置填充颜色。
- ctx.fill()方法:填充路径。
- requestAnimationFrame()方法:浏览器调用下一帧动画之前会执行该方法中的回调函数,可以实现平滑的动画效果。
阅读全文
相关推荐
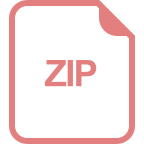
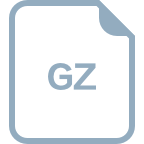
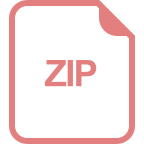















