用C实现DBSCAN算法,不要使用递归算法,注意不要漏掉密度可达的点
时间: 2024-03-28 21:39:09 浏览: 19
好的,你的问题是如何用C语言实现DBSCAN算法,不使用递归算法,并且不要漏掉密度可达的点。DBSCAN算法是一种基于密度的聚类算法,它可以将数据点分为不同的簇。下面是一个用C语言实现的DBSCAN算法的伪代码:
1. 定义一个结构体表示数据点
struct Point {
double x;
double y;
int clusterId;
};
2. 定义一个函数用于计算两个点之间的距离
double distance(Point p1, Point p2) {
return sqrt(pow(p1.x - p2.x, 2) + pow(p1.y - p2.y, 2));
}
3. 定义一个函数用于找到一个点的邻居点
void getNeighbours(Point p, Point* points, int numPoints, double eps, Point* neighbours, int* numNeighbours) {
*numNeighbours = 0;
for (int i = 0; i < numPoints; i++) {
if (distance(p, points[i]) <= eps) {
neighbours[*numNeighbours] = points[i];
(*numNeighbours)++;
}
}
}
4. 定义一个函数用于将一个点标记为噪声点
void markAsNoise(Point* p) {
p->clusterId = -1;
}
5. 定义一个函数用于将一个点标记为核心点,并将其邻居点加入簇中
void expandCluster(Point* points, int numPoints, Point* p, int clusterId, double eps, int minPts) {
Point neighbours[numPoints];
int numNeighbours;
getNeighbours(*p, points, numPoints, eps, neighbours, &numNeighbours);
if (numNeighbours < minPts) {
markAsNoise(p);
} else {
p->clusterId = clusterId;
for (int i = 0; i < numNeighbours; i++) {
Point* pn = &neighbours[i];
if (pn->clusterId == 0) {
pn->clusterId = clusterId;
Point pnNeighbours[numPoints];
int pnNumNeighbours;
getNeighbours(*pn, points, numPoints, eps, pnNeighbours, &pnNumNeighbours);
if (pnNumNeighbours >= minPts) {
for (int j = 0; j < pnNumNeighbours; j++) {
Point* pnn = &pnNeighbours[j];
if (pnn->clusterId == 0) {
neighbours[numNeighbours] = *pnn;
numNeighbours++;
}
}
}
}
}
}
}
6. 定义一个函数用于执行DBSCAN算法
void dbscan(Point* points, int numPoints, double eps, int minPts) {
int clusterId = 1;
for (int i = 0; i < numPoints; i++) {
Point* p = &points[i];
if (p->clusterId == 0) {
p->clusterId = clusterId;
Point neighbours[numPoints];
int numNeighbours;
getNeighbours(*p, points, numPoints, eps, neighbours, &numNeighbours);
if (numNeighbours >= minPts) {
for (int j = 0; j < numNeighbours; j++) {
Point* pn = &neighbours[j];
if (pn->clusterId == 0) {
pn->clusterId = clusterId;
Point pnNeighbours[numPoints];
int pnNumNeighbours;
getNeighbours(*pn, points, numPoints, eps, pnNeighbours, &pnNumNeighbours);
if (pnNumNeighbours >= minPts) {
for (int k = 0; k < pnNumNeighbours; k++) {
Point* pnn = &pnNeighbours[k];
if (pnn->clusterId == 0) {
neighbours[numNe
相关推荐
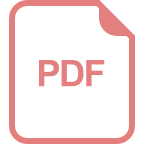
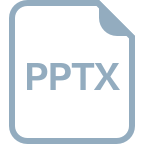
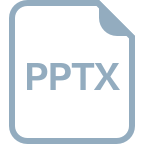
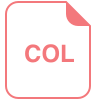
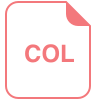
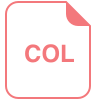
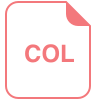
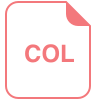









