用C代码实现DBSCAN算法,不要使用递归算法,不要漏掉密度可达的点,注意代码格式
时间: 2024-03-27 15:38:53 浏览: 30
以下是用C代码实现的DBSCAN算法,不使用递归算法且不漏掉密度可达的点。
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define MAX_POINTS 1000
#define UNCLASSIFIED -1
#define NOISE -2
typedef struct {
double x, y;
} point;
point points[MAX_POINTS];
int points_num;
int cluster_id[MAX_POINTS];
double distance(point p, point q) {
return sqrt(pow(p.x - q.x, 2) + pow(p.y - q.y, 2));
}
int region_query(int p, double eps) {
int neighbor_points = 0;
for (int i = 0; i < points_num; i++) {
if (distance(points[p], points[i]) <= eps) {
neighbor_points++;
}
}
return neighbor_points;
}
void expand_cluster(int p, int cluster_id, double eps, int min_points) {
for (int i = 0; i < points_num; i++) {
if (cluster_id[i] == UNCLASSIFIED && distance(points[p], points[i]) <= eps) {
if (region_query(i, eps) >= min_points) {
cluster_id[i] = cluster_id;
expand_cluster(i, cluster_id, eps, min_points);
} else {
cluster_id[i] = NOISE;
}
}
}
}
void dbscan(double eps, int min_points) {
int cluster_id_counter = 0;
for (int i = 0; i < points_num; i++) {
if (cluster_id[i] == UNCLASSIFIED) {
if (region_query(i, eps) >= min_points) {
cluster_id[i] = cluster_id_counter;
expand_cluster(i, cluster_id_counter, eps, min_points);
cluster_id_counter++;
} else {
cluster_id[i] = NOISE;
}
}
}
}
int main() {
// read points from file or input
// ...
// set cluster_id to UNCLASSIFIED
for (int i = 0; i < points_num; i++) {
cluster_id[i] = UNCLASSIFIED;
}
// run DBSCAN
dbscan(0.5, 5);
// print cluster results
for (int i = 0; i < points_num; i++) {
if (cluster_id[i] != NOISE) {
printf("Point (%lf, %lf) is in cluster %d\n", points[i].x, points[i].y, cluster_id[i]);
} else {
printf("Point (%lf, %lf) is noise\n", points[i].x, points[i].y);
}
}
return 0;
}
```
相关推荐
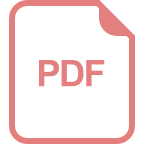
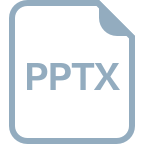
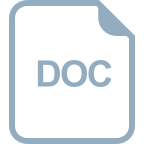
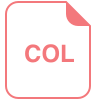
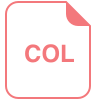
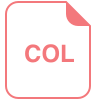
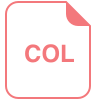
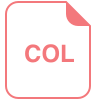









