用c语言编写迷宫小游戏
时间: 2023-10-25 21:06:32 浏览: 156
好的,我可以为您提供一个简单的迷宫小游戏的C语言代码示例,您可以根据需要进行修改和扩展。
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define ROWS 10
#define COLS 10
void createMaze(int maze[][COLS], int rows, int cols);
void printMaze(int maze[][COLS], int rows, int cols);
int solveMaze(int maze[][COLS], int rows, int cols, int row, int col);
int main()
{
int maze[ROWS][COLS];
srand(time(NULL));
createMaze(maze, ROWS, COLS);
printMaze(maze, ROWS, COLS);
if (solveMaze(maze, ROWS, COLS, 0, 0))
printf("Congratulations! You have solved the maze.\n");
else
printf("Sorry, you failed to solve the maze.\n");
return 0;
}
// 创建迷宫
void createMaze(int maze[][COLS], int rows, int cols)
{
int i, j;
for (i = 0; i < rows; i++) {
for (j = 0; j < cols; j++) {
maze[i][j] = rand() % 2;
}
}
maze[0][0] = 0; // 入口
maze[rows - 1][cols - 1] = 0; // 出口
}
// 打印迷宫
void printMaze(int maze[][COLS], int rows, int cols)
{
int i, j;
for (i = 0; i < rows; i++) {
for (j = 0; j < cols; j++) {
if (maze[i][j] == 0)
printf(" ");
else
printf("# ");
}
printf("\n");
}
}
// 解迷宫
int solveMaze(int maze[][COLS], int rows, int cols, int row, int col)
{
if (row < 0 || row >= rows || col < 0 || col >= cols) // 越界
return 0;
if (maze[row][col] == 1) // 障碍
return 0;
if (row == rows - 1 && col == cols - 1) // 到达出口
return 1;
maze[row][col] = 1; // 标记已经访问过
if (solveMaze(maze, rows, cols, row - 1, col)) // 向上走
return 1;
if (solveMaze(maze, rows, cols, row + 1, col)) // 向下走
return 1;
if (solveMaze(maze, rows, cols, row, col - 1)) // 向左走
return 1;
if (solveMaze(maze, rows, cols, row, col + 1)) // 向右走
return 1;
maze[row][col] = 0; // 回溯
return 0;
}
```
该迷宫小游戏使用了递归算法来解决迷宫问题。在迷宫创建时,随机生成了一些障碍物,并标记了入口和出口。在解迷宫时,从入口开始,每次尝试向上、下、左、右四个方向走,如果可以走通,则递归进入下一层,直到到达出口或走不通为止。如果走不通,则回溯到上一层,尝试其他方向。最终,如果找到了一条通路,则返回1,否则返回0。
阅读全文
相关推荐
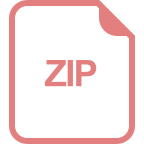
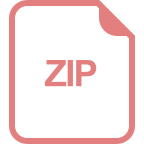
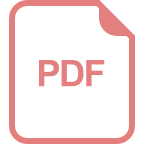















