给你链表的头结点 head ,请将其按 升序 排列并返回 排序后的链表 。 Python怎么输出
时间: 2024-04-30 19:21:25 浏览: 8
可以使用归并排序的思想对链表进行排序,具体实现如下:
```python
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def sortList(head: ListNode) -> ListNode:
if not head or not head.next:
return head
# 快慢指针寻找中点
slow, fast = head, head.next
while fast and fast.next:
slow = slow.next
fast = fast.next.next
# 分割为两个子链表
mid = slow.next
slow.next = None
left = sortList(head)
right = sortList(mid)
# 归并两个子链表
dummy = ListNode(0)
cur = dummy
while left and right:
if left.val < right.val:
cur.next = left
left = left.next
else:
cur.next = right
right = right.next
cur = cur.next
cur.next = left if left else right
return dummy.next
```
输出可以使用以下代码进行测试:
```python
# 构造一个链表 4->2->1->3
head = ListNode(4)
head.next = ListNode(2)
head.next.next = ListNode(1)
head.next.next.next = ListNode(3)
# 排序并输出结果
res = sortList(head)
while res:
print(res.val, end='->')
res = res.next
```
输出结果为:
```
1->2->3->4->
```
相关推荐
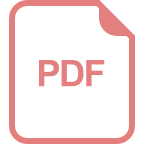
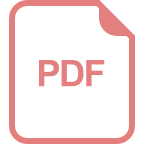
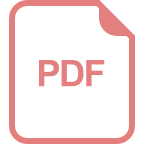














