对有向图运用邻接表存储并进行深度优先遍历,c代码
时间: 2023-12-16 07:08:36 浏览: 38
下面是一个有向图使用邻接表存储并进行深度优先遍历的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_VERTICES 100
typedef struct Node {
int vertex;
struct Node* next;
} Node;
Node* graph[MAX_VERTICES] = { NULL };
int visited[MAX_VERTICES] = { 0 };
void add_edge(int u, int v) {
Node* new_node = (Node*)malloc(sizeof(Node));
new_node->vertex = v;
new_node->next = graph[u];
graph[u] = new_node;
}
void dfs(int v) {
visited[v] = 1;
printf("%d ", v);
Node* node = graph[v];
while (node) {
int next = node->vertex;
if (!visited[next]) {
dfs(next);
}
node = node->next;
}
}
int main() {
int vertices, edges;
printf("Enter the number of vertices and edges: ");
scanf("%d%d", &vertices, &edges);
printf("Enter the edges (from to): ");
for (int i = 0; i < edges; i++) {
int u, v;
scanf("%d%d", &u, &v);
add_edge(u, v);
}
int start;
printf("Enter the starting vertex: ");
scanf("%d", &start);
printf("DFS traversal: ");
dfs(start);
printf("\n");
return 0;
}
```
在该代码中,我们使用了一个`Node`结构体来表示邻接表中的每个节点。`add_edge()`函数用于向邻接表中添加一条边。`dfs()`函数是递归实现的深度优先遍历函数,它从给定的起始节点开始遍历整个图,并使用`visited`数组来跟踪哪些节点已经被访问过了。最后,`main()`函数读取输入数据,构建邻接表,然后调用`dfs()`函数以遍历整个图。
相关推荐
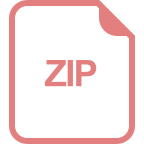
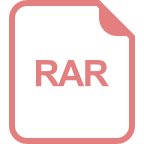
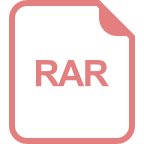
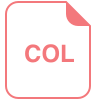
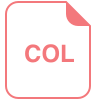
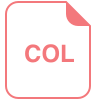
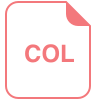
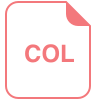









