Severity Code Description Project File Line Suppression State Error CS0263 Partial declarations of 'LevelControl' must not specify different base classes New C:\Users\huahuil\Source\Repos\JoeMihhh\New_LearnWPF\New\View\LevelControl.xaml.cs 20 Active // using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows; using System.Windows.Controls; using System.Windows.Data; using System.Windows.Documents; using System.Windows.Input; using System.Windows.Media; using System.Windows.Media.Imaging; using System.Windows.Shapes; namespace New.View { /// <summary> /// Interaction logic for LevelControl.xaml /// </summary> public partial class LevelControl : UserControl { public LevelControl() { InitializeComponent(); } private void ComboBox_SelectionChanged(object sender, SelectionChangedEventArgs e) { //The if prevents taking any action when the *first* item is selected, which is done on start up. if (e.RemovedItems.Count > 0) { MessageBox.Show("Dropdown list used to select: " + e.AddedItems[0]); } //Consider assigning genders to the Employee objects populated from the HRIS database (which do not have a gender), //then use code similar to that in MainWindow to gain access to the Boss object and request that it filter //the list by the selected Gender. } } }
时间: 2024-02-14 20:04:35 浏览: 184
这个错误表示部分类的基类不一致。在你的示例中,LevelControl 的两个部分类指定的基类不同,这是不能被允许的。
为了解决这个问题,你需要确保所有部分类的基类相同。在你的情况下,LevelControl.xaml.cs 中的部分类应该与 LevelControl.xaml 中的部分类使用相同的基类。
例如,如果 LevelControl.xaml 中的类定义如下:
```
public partial class LevelControl : UserControl
{
// Class implementation here
}
```
那么,LevelControl.xaml.cs 中的类定义也应该相同:
```
public partial class LevelControl : UserControl
{
// Class implementation here
}
```
这样,两个部分类的基类就是相同的,编译器就不会报错了。
相关问题
how to solve Severity Code Description Project File Line Suppression State Error C3861 'glutCreateWindow': identifier not found OpenGL C:\Users\Asus\OpenGL\OpenGL\test1.cpp 18
The error message you're seeing indicates that the `glutCreateWindow` function was not found by the compiler. This typically means that the appropriate header file has not been included or the necessary libraries have not been linked.
To resolve this issue, you should ensure that you have included the necessary header files and linked the appropriate libraries. Specifically, you need to include the `glut.h` header file and link against the `glut32.lib` library.
Here's an updated version of the example code with the necessary changes:
```
#include <GL/glut.h>
void display()
{
glClear(GL_COLOR_BUFFER_BIT);
glBegin(GL_POLYGON);
glVertex2f(-0.5, -0.5);
glVertex2f(-0.5, 0.5);
glVertex2f(0.5, 0.5);
glVertex2f(0.5, -0.5);
glEnd();
glFlush();
}
int main(int argc, char** argv)
{
glutInit(&argc, argv);
glutCreateWindow("OpenGL Test");
glutDisplayFunc(display);
glutMainLoop();
return 0;
}
```
Make sure that you have the necessary libraries and header files installed and linked correctly.
Severity Code Description Project File Line Suppression State Error (active) E1696 cannot open source file "graphics.h" MFCApplication2 D:\vs project\MFCApplication2\MFCApplication2\MyCircle.cpp 2
这个错误通常是因为编译器找不到 `graphics.h` 头文件而导致的。这个头文件是 Borland Graphics Interface (BGI) 图形库的一部分,这个库通常在 Turbo C++ 和 Borland C++ 中使用。
如果你在 Visual Studio 中使用 Bresenham 算法绘制圆形,可以使用 Windows GDI (Graphics Device Interface) 或者其他图形库,例如 OpenGL 和 DirectX。这些库都可以用于在屏幕上绘制图形,而且在 Windows 平台上都有完整的支持。
下面是一个使用 Windows GDI 绘制圆形的示例代码:
```c++
#include <iostream>
#include <windows.h>
using namespace std;
LRESULT CALLBACK WindowProc(HWND hwnd, UINT uMsg, WPARAM wParam, LPARAM lParam);
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, LPSTR lpCmdLine, int nCmdShow)
{
const char CLASS_NAME[] = "MyWindowClass";
WNDCLASS wc = {};
wc.lpfnWndProc = WindowProc;
wc.hInstance = hInstance;
wc.lpszClassName = CLASS_NAME;
RegisterClass(&wc);
HWND hwnd = CreateWindowEx(
0,
CLASS_NAME,
"My Window",
WS_OVERLAPPEDWINDOW,
CW_USEDEFAULT, CW_USEDEFAULT, CW_USEDEFAULT, CW_USEDEFAULT,
NULL,
NULL,
hInstance,
NULL
);
if (hwnd == NULL)
{
return 0;
}
ShowWindow(hwnd, nCmdShow);
MSG msg = {};
while (GetMessage(&msg, NULL, 0, 0))
{
TranslateMessage(&msg);
DispatchMessage(&msg);
}
return 0;
}
LRESULT CALLBACK WindowProc(HWND hwnd, UINT uMsg, WPARAM wParam, LPARAM lParam)
{
switch (uMsg)
{
case WM_PAINT:
{
PAINTSTRUCT ps;
HDC hdc = BeginPaint(hwnd, &ps);
int x0 = ps.rcPaint.right / 2;
int y0 = ps.rcPaint.bottom / 2;
int r = 100;
HPEN hPen = CreatePen(PS_SOLID, 2, RGB(255, 255, 255));
HGDIOBJ hOldPen = SelectObject(hdc, hPen);
Ellipse(hdc, x0 - r, y0 - r, x0 + r, y0 + r);
SelectObject(hdc, hOldPen);
DeleteObject(hPen);
EndPaint(hwnd, &ps);
}
break;
case WM_DESTROY:
PostQuitMessage(0);
break;
default:
return DefWindowProc(hwnd, uMsg, wParam, lParam);
}
return 0;
}
```
这个程序创建了一个 Windows 窗口,并在窗口中心绘制一个半径为 100 的圆形。在 `WindowProc` 函数中,我们处理 `WM_PAINT` 消息,并使用 `Ellipse` 函数绘制圆形。`BeginPaint` 和 `EndPaint` 函数用于获取和释放绘图设备句柄,而 `CreatePen` 和 `SelectObject` 函数用于创建和选择画笔对象,以便绘制白色的圆形。
阅读全文
相关推荐
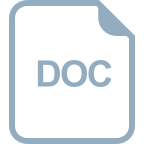
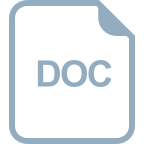
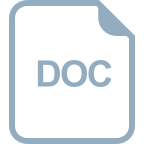


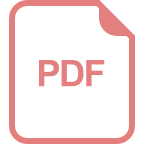
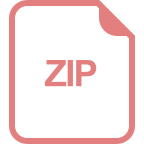









