用c语言写一个具有过程回放功能的五子棋游戏。
时间: 2024-03-23 07:38:36 浏览: 65
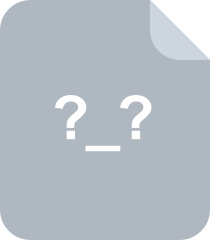
一个C语言的五子棋游戏
以下是用C语言写一个具有过程回放功能的五子棋游戏的代码示例,代码中包含了注释和说明,方便理解和参考:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#include <conio.h>
#include <windows.h>
#define BOARD_SIZE 15 // 棋盘大小
#define EMPTY ' ' // 空格
#define PLAYER1 'X' // 玩家1用X表示
#define PLAYER2 'O' // 玩家2用O表示
// 定义坐标结构体,表示棋子的位置
typedef struct {
int row; // 行
int col; // 列
} Position;
// 定义棋盘数组,用于存储棋子的位置
char board[BOARD_SIZE][BOARD_SIZE];
// 定义当前玩家,初始为玩家1
char currentPlayer = PLAYER1;
// 定义下棋的计数器,用于记录已下的棋子数
int moveCount = 0;
// 定义存储棋谱的数组,用于实现回放功能
Position moveList[BOARD_SIZE * BOARD_SIZE];
int moveIndex = 0;
// 初始化棋盘,将所有位置都赋值为空格
void initBoard() {
for (int i = 0; i < BOARD_SIZE; i++) {
for (int j = 0; j < BOARD_SIZE; j++) {
board[i][j] = EMPTY;
}
}
}
// 绘制棋盘
void drawBoard() {
system("cls"); // 清屏
printf(" "); // 打印列号
for (int i = 0; i < BOARD_SIZE; i++) {
printf("%d ", i + 1);
}
printf("\n");
for (int i = 0; i < BOARD_SIZE; i++) {
printf("%2d", i + 1); // 打印行号
for (int j = 0; j < BOARD_SIZE; j++) {
printf("%c|", board[i][j]); // 打印棋盘格子
}
printf("\n");
for (int j = 0; j < BOARD_SIZE; j++) {
printf("--"); // 打印分隔线
}
printf("\n");
}
}
// 判断是否有一方获胜
bool checkWin(char player) {
// 横向判断
for (int i = 0; i < BOARD_SIZE; i++) {
for (int j = 0; j < BOARD_SIZE - 4; j++) {
if (board[i][j] == player && board[i][j + 1] == player &&
board[i][j + 2] == player && board[i][j + 3] == player &&
board[i][j + 4] == player) {
return true;
}
}
}
// 纵向判断
for (int i = 0; i < BOARD_SIZE - 4; i++) {
for (int j = 0; j < BOARD_SIZE; j++) {
if (board[i][j] == player && board[i + 1][j] == player &&
board[i + 2][j] == player && board[i + 3][j] == player &&
board[i + 4][j] == player) {
return true;
}
}
}
// 左上到右下判断
for (int i = 0; i < BOARD_SIZE - 4; i++) {
for (int j = 0; j < BOARD_SIZE - 4; j++) {
if (board[i][j] == player && board[i + 1][j + 1] == player &&
board[i + 2][j + 2] == player && board[i + 3][j + 3] == player &&
board[i + 4][j + 4] == player) {
return true;
}
}
}
// 右上到左下判断
for (int i = 0; i < BOARD_SIZE - 4; i++) {
for (int j = 4; j < BOARD_SIZE; j++) {
if (board[i][j] == player && board[i + 1][j - 1] == player &&
board[i + 2][j - 2] == player && board[i + 3][j - 3] == player &&
board[i + 4][j - 4] == player) {
return true;
}
}
}
return false;
}
// 判断是否平局
bool checkTie() {
return moveCount == BOARD_SIZE * BOARD_SIZE;
}
// 切换玩家
void switchPlayer() {
if (currentPlayer == PLAYER1) {
currentPlayer = PLAYER2;
} else {
currentPlayer = PLAYER1;
}
}
// 保存棋谱
void saveMove(Position pos) {
moveList[moveIndex++] = pos;
}
// 回放棋谱
void replayMoves() {
for (int i = 0; i < moveIndex; i++) {
Position pos = moveList[i];
board[pos.row][pos.col] = i % 2 == 0 ? PLAYER1 : PLAYER2;
drawBoard();
Sleep(500);
}
}
// 主函数
int main() {
initBoard(); // 初始化棋盘
drawBoard(); // 绘制棋盘
while (true) {
// 等待玩家输入
printf("Player %c's turn, please enter the row and column (e.g. 1 1): ", currentPlayer);
int row, col;
scanf("%d %d", &row, &col);
fflush(stdin); // 清空缓冲区
row--; // 行号和列号从1开始,需要减1
col--;
// 判断输入是否合法
if (row < 0 || row >= BOARD_SIZE || col < 0 || col >= BOARD_SIZE) {
printf("Invalid position, please try again.\n");
continue;
}
if (board[row][col] != EMPTY) {
printf("The position has been occupied, please try again.\n");
continue;
}
// 下棋
board[row][col] = currentPlayer;
moveCount++;
Position pos = {row, col};
saveMove(pos);
drawBoard();
// 判断是否获胜或平局
if (checkWin(currentPlayer)) {
printf("Player %c wins!\n", currentPlayer);
break;
}
if (checkTie()) {
printf("Tie!\n");
break;
}
// 切换玩家
switchPlayer();
}
// 回放棋谱
printf("Do you want to replay the game? (y/n): ");
char choice;
scanf("%c", &choice);
if (choice == 'y' || choice == 'Y') {
replayMoves();
}
return 0;
}
```
以上代码实现了一个具有过程回放功能的五子棋游戏,通过保存棋谱和回放棋谱的方式实现了回放功能。代码中还包含了绘制棋盘、下棋、判断胜负等基本功能的实现。可以根据需要进行修改和优化。
阅读全文
相关推荐
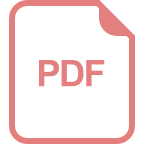





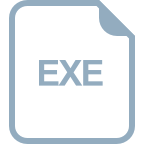
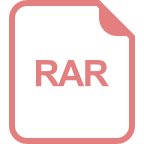
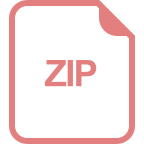
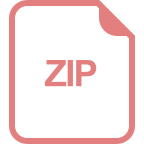
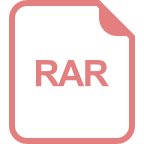
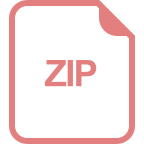
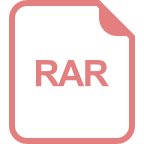
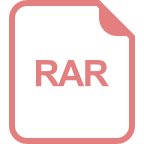
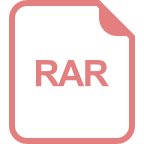
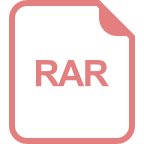
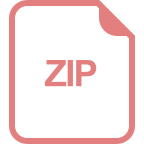