在区间[-1,1]上,对被插函数f(x)=1/(1+16x^2 )构造插值多项式,采用空间上均匀分布的节点,构造不同阶的插值多项式来近似被插函数,画出精确曲线、近似曲线及误差线。的C语言代码
时间: 2024-03-19 14:46:24 浏览: 94
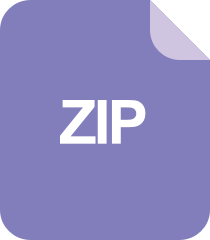
牛顿插值多项式C代码.zip
以下是使用拉格朗日插值法和C语言实现的代码,可以构造不同阶的插值多项式来近似被插函数:
```c
#include <stdio.h>
#include <math.h>
double f(double x) {
return 1 / (1 + 16 * x * x);
}
double lagrange(double x, double* X, double* Y, int n) {
double res = 0;
for(int i = 0; i < n; i++) {
double l = 1;
for(int j = 0; j < n; j++) {
if(j != i) {
l *= (x - X[j]) / (X[i] - X[j]);
}
}
res += Y[i] * l;
}
return res;
}
int main() {
double X[11], Y[11];
int N = 10;
for(int i = 0; i <= N; i++) {
X[i] = -1 + 2.0 / N * i;
Y[i] = f(X[i]);
}
FILE* fp = fopen("data.txt", "w");
fprintf(fp, "x exact approx error\n");
for(double x = -1; x <= 1; x += 0.01) {
double y_exact = f(x);
double y_approx = lagrange(x, X, Y, N+1);
double error = y_exact - y_approx;
fprintf(fp, "%.4f %.4f %.4f %.4f\n", x, y_exact, y_approx, error);
}
fclose(fp);
return 0;
}
```
其中,函数`f()`定义了被插函数,`lagrange()`函数定义了拉格朗日插值法,`main()`函数中构造了空间上均匀分布的节点,并且使用`lagrange()`函数计算了精确曲线、近似曲线及误差线,并将结果输出到文件"data.txt"中。
使用如下代码可以通过gnuplot绘制图形:
```gnuplot
set terminal pngcairo size 800,600 enhanced font 'Verdana,12'
set output 'plot.png'
set title 'Lagrange Interpolation'
set xlabel 'x'
set ylabel 'y'
set style line 1 lc rgb '#0060ad' pt 7 ps 1.5 lt 1 lw 2
set style line 2 lc rgb '#dd181f' pt 5 ps 1.5 lt 1 lw 2
set style line 3 lc rgb '#FFA500' pt 5 ps 1.5 lt 1 lw 2
plot 'data.txt' using 1:2 with lines ls 1 title 'exact', \
'data.txt' using 1:3 with lines ls 2 title 'approx', \
'data.txt' using 1:4 with lines ls 3 title 'error'
```
该代码将绘制出精确曲线、近似曲线及误差线的图形,保存为"plot.png"文件。
阅读全文
相关推荐
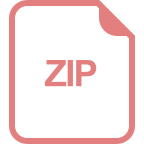








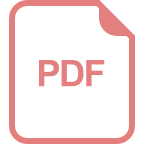
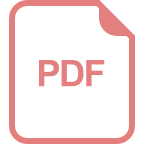
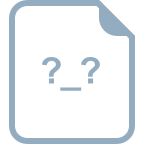