c语言图片添加水印程序,[求助]C语言 bmp文件加上水印
时间: 2023-12-02 11:03:06 浏览: 189
以下是一个简单的 C 语言示例程序,可以将 BMP 文件添加水印:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#pragma pack(push, 1)
typedef struct {
unsigned short int bfType;
unsigned int bfSize;
unsigned short int bfReserved1;
unsigned short int bfReserved2;
unsigned int bfOffBits;
} BITMAPFILEHEADER;
typedef struct {
unsigned int biSize;
int biWidth;
int biHeight;
unsigned short int biPlanes;
unsigned short int biBitCount;
unsigned int biCompression;
unsigned int biSizeImage;
int biXPelsPerMeter;
int biYPelsPerMeter;
unsigned int biClrUsed;
unsigned int biClrImportant;
} BITMAPINFOHEADER;
#pragma pack(pop)
int main(int argc, char *argv[]) {
if (argc < 4) {
printf("Usage: %s input.bmp output.bmp watermark\n", argv[0]);
return 1;
}
char *input_file = argv[1];
char *output_file = argv[2];
char *watermark = argv[3];
FILE *input = fopen(input_file, "rb");
if (input == NULL) {
printf("Failed to open input file: %s\n", input_file);
return 1;
}
BITMAPFILEHEADER file_header;
BITMAPINFOHEADER info_header;
fread(&file_header, sizeof(BITMAPFILEHEADER), 1, input);
fread(&info_header, sizeof(BITMAPINFOHEADER), 1, input);
if (file_header.bfType != 0x4D42 || info_header.biBitCount != 24) {
printf("Invalid BMP file format\n");
fclose(input);
return 1;
}
int width = info_header.biWidth;
int height = info_header.biHeight;
int padding = (4 - ((width * 3) % 4)) % 4;
char *image_data = (char *) malloc(sizeof(char) * width * height * 3);
fread(image_data, sizeof(char), width * height * 3, input);
fclose(input);
int watermark_length = strlen(watermark);
int i, j, k;
for (i = 0; i < width; i++) {
for (j = 0; j < height; j++) {
int index = j * width + i;
int r = image_data[index * 3 + 2];
int g = image_data[index * 3 + 1];
int b = image_data[index * 3];
if (i < watermark_length) {
char c = watermark[i];
int value = (int) c;
for (k = 0; k < 8; k++) {
int bit = (value >> (7 - k)) & 1;
if (bit == 1) {
if (j < height - 10) {
image_data[index * 3 + 2] = 255;
image_data[index * 3 + 1] = 0;
image_data[index * 3] = 0;
}
}
index += width;
}
}
if (j == height - 1 && i == width - 1) {
printf("Finished adding watermark\n");
}
}
}
FILE *output = fopen(output_file, "wb");
if (output == NULL) {
printf("Failed to create output file: %s\n", output_file);
free(image_data);
return 1;
}
fwrite(&file_header, sizeof(BITMAPFILEHEADER), 1, output);
fwrite(&info_header, sizeof(BITMAPINFOHEADER), 1, output);
fwrite(image_data, sizeof(char), width * height * 3, output);
fclose(output);
free(image_data);
printf("Successfully saved watermarked image to %s\n", output_file);
return 0;
}
```
这个程序需要三个参数:输入 BMP 文件名、输出 BMP 文件名和水印字符串。例如,如果要将名为 "input.bmp" 的 BMP 文件添加水印 "Hello, world!" 并保存为 "output.bmp" 文件,则可以执行以下命令:
```
./watermark input.bmp output.bmp "Hello, world!"
```
请注意,此程序仅适用于 24 位色深的 BMP 文件。如果 BMP 文件的色深不是 24 位,则需要相应地修改程序。
阅读全文
相关推荐
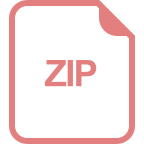
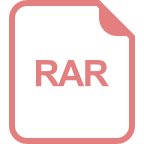
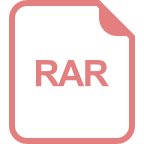
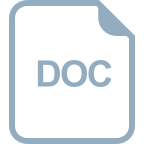
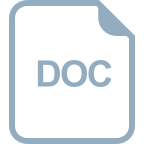







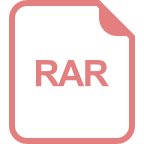
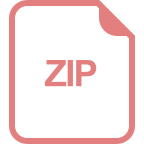