写一个C++程序 实现迷宫
时间: 2023-06-04 20:02:57 浏览: 59
很高兴为您回答这个问题!以下是一个简单的迷宫求解程序:
```c
#include <stdio.h>
// 定义迷宫大小和路径
#define ROW_SIZE 10
#define COL_SIZE 10
int maze[ROW_SIZE][COL_SIZE] = {
{1,1,1,1,1,1,1,1,1,1},
{0,0,1,0,0,0,1,0,1,1},
{1,0,1,0,1,0,1,0,1,1},
{1,0,1,0,1,0,0,0,0,1},
{1,0,1,1,1,1,1,1,0,1},
{1,0,1,0,0,0,0,0,0,1},
{1,0,1,0,1,1,1,1,0,1},
{1,0,0,0,0,0,0,0,0,1},
{1,1,1,1,1,1,1,1,1,1},
{0,0,0,0,0,0,0,0,0,1}
};
int path[ROW_SIZE][COL_SIZE];
// 定义方向
#define EAST 0
#define NORTH 1
#define WEST 2
#define SOUTH 3
// 定义堆栈
#define MAX_STACK_SIZE 100
int stack[MAX_STACK_SIZE];
int top = -1;
void push(int item) {
if (top >= MAX_STACK_SIZE - 1) {
printf("stack overflow\n");
return;
}
stack[++top] = item;
}
int pop() {
if (top < 0) {
printf("stack underflow\n");
return -1;
}
return stack[top--];
}
int is_empty() {
return top < 0;
}
int get_direction(int r, int c, int direction) {
switch (direction) {
case EAST: return maze[r][c + 1];
case NORTH: return maze[r - 1][c];
case WEST: return maze[r][c - 1];
case SOUTH: return maze[r + 1][c];
}
}
void print_path() {
printf("Path:\n");
for (int i = 0; i < ROW_SIZE; i++) {
for (int j = 0; j < COL_SIZE; j++) {
printf("%d ", path[i][j]);
}
printf("\n");
}
}
void solve_maze(int r, int c, int direction) {
push(direction);
while (!is_empty()) {
direction = pop();
path[r][c] = 1;
if (r == ROW_SIZE - 1 && c == COL_SIZE - 1) {
print_path();
return;
}
for (int i = direction + 1; i < direction + 5; i++) {
int new_direction = i % 4;
if (get_direction(r, c, new_direction) == 0) {
switch (new_direction) {
case EAST: c++; break;
case NORTH: r--; break;
case WEST: c--; break;
case SOUTH: r++; break;
}
push(new_direction);
break;
}
}
}
printf("No solution found\n");
}
int main() {
solve_maze(0, 0, EAST);
return 0;
}
```
该程序使用堆栈和回溯来解决迷宫问题。迷宫的大小由 `ROW_SIZE` 和 `COL_SIZE` 定义,路径由 `maze` 数组表示。程序搜索所有可能的路径,打印出正确路径。
相关推荐
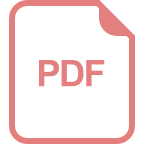
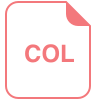













