编写程序模拟掷骰子的游戏(两个骰子)。每局游戏的规则如下: 第一次掷的时候,如 果点数之和为 7 或 11 则获胜;如果点数之和为2、3或12则落败;其他情况下的点数之和称为"目标",游戏继续。在后续的投掷中,如果玩家再次掷出"目标"点数则获胜,掷出7则落败,其他情况都忽略,游戏继续进行。每局游戏结束时,程序询问用户是否再玩一次,如果用 户输入的回答不是 y 或 Y ,程序会显示胜败的次数然后终止。用C语言实现
时间: 2024-03-22 07:37:50 浏览: 78
好的,以下是 C 语言实现的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
int main() {
int win = 0, lose = 0; // 记录胜利和失败的次数
char choice; // 记录用户是否继续游戏的选择
srand(time(NULL)); // 初始化随机数种子
do {
int point = 0, target = 0, sum = 0;
// 第一次掷骰子
sum = rand() % 6 + 1 + rand() % 6 + 1;
if (sum == 7 || sum == 11) {
printf("You win!\n");
win++;
} else if (sum == 2 || sum == 3 || sum == 12) {
printf("You lose!\n");
lose++;
} else {
target = sum;
printf("Target is %d\n", target);
// 后续掷骰子,直到获胜或落败
do {
sum = rand() % 6 + 1 + rand() % 6 + 1;
if (sum == target) {
printf("You win!\n");
win++;
break;
} else if (sum == 7) {
printf("You lose!\n");
lose++;
break;
}
} while (1);
}
// 询问用户是否继续游戏
printf("Do you want to play again? (y/n) ");
scanf(" %c", &choice);
} while (choice == 'y' || choice == 'Y');
// 显示胜利和失败的次数
printf("Wins: %d\n", win);
printf("Loses: %d\n", lose);
return 0;
}
```
以上代码使用了 `srand` 函数和 `rand` 函数来生成随机数,其中 `srand` 函数用于初始化随机数种子,`rand` 函数用于生成一个介于 1 到 6 之间的随机数,模拟掷骰子的过程。程序使用了 `do-while` 循环来实现游戏的连续进行,直到用户选择不再继续游戏为止。
阅读全文
相关推荐






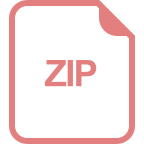
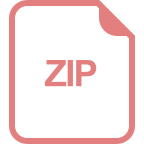
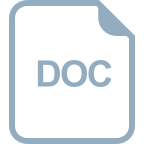

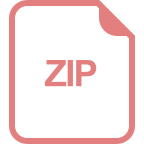
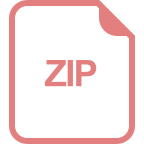