分页功能实现前端vue+java后端代码
时间: 2023-07-30 21:10:21 浏览: 122
前端 Vue.js 代码:
```
<template>
<div>
<table>
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Price</th>
</tr>
</thead>
<tbody>
<tr v-for="product in products" :key="product.id">
<td>{{ product.id }}</td>
<td>{{ product.name }}</td>
<td>{{ product.price }}</td>
</tr>
</tbody>
</table>
<el-pagination
v-if="total > 0"
:page-size="pageSize"
:total="total"
:current-page="currentPage"
@current-change="handlePageChange"
/>
</div>
</template>
<script>
import axios from 'axios'
export default {
data() {
return {
products: [],
currentPage: 1,
pageSize: 10,
total: 0
}
},
mounted() {
this.getProducts()
},
methods: {
getProducts() {
axios.get('/api/products', {
params: {
page: this.currentPage,
pageSize: this.pageSize
}
}).then(res => {
this.products = res.data.list
this.total = res.data.total
})
},
handlePageChange(page) {
this.currentPage = page
this.getProducts()
}
}
}
</script>
```
后端 Java Spring Boot 代码:
```
@RestController
@RequestMapping("/api")
public class ProductController {
@Autowired
private ProductService productService;
@GetMapping("/products")
public PageResult<Product> getProducts(@RequestParam Integer page, @RequestParam Integer pageSize) {
return productService.getProducts(page, pageSize);
}
}
```
```
@Service
public class ProductServiceImpl implements ProductService {
@Autowired
private ProductRepository productRepository;
@Override
public PageResult<Product> getProducts(Integer page, Integer pageSize) {
Sort sort = Sort.by(Sort.Direction.DESC, "id");
Pageable pageable = PageRequest.of(page - 1, pageSize, sort);
Page<Product> productPage = productRepository.findAll(pageable);
List<Product> productList = productPage.getContent();
long total = productPage.getTotalElements();
return new PageResult<>(productList, total);
}
}
```
```
public interface ProductService {
PageResult<Product> getProducts(Integer page, Integer pageSize);
}
```
```
public interface ProductRepository extends JpaRepository<Product, Long> {
}
```
以上是一个简单的Vue.js + Java Spring Boot实现分页功能的示例,具体实现还需要根据业务需求进行调整。
阅读全文
相关推荐
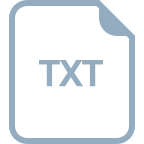
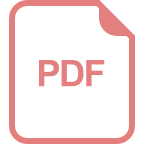


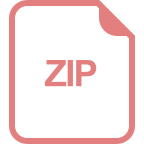
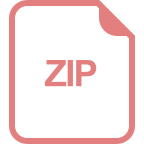
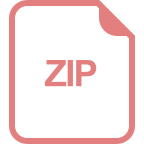
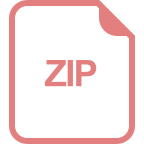
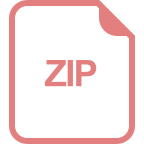
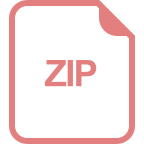
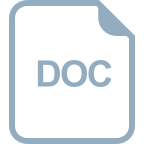
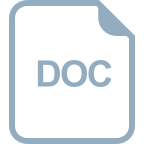
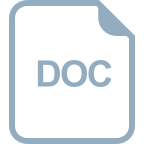
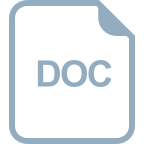
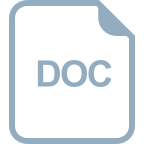


