帮我用c语言写一个中缀表达式转换为后缀表达式的算法
时间: 2023-05-25 16:02:27 浏览: 114
A:
中缀表达式转换为后缀表达式需要用到栈作为辅助数据结构,具体的算法步骤如下:
1. 从左到右扫描中缀表达式的每个字符
2. 如果是操作数,则直接输出到后缀表达式中
3. 如果是左括号,将其压入栈中
4. 如果是右括号,将栈中左括号之后的操作符全部弹出并输出到后缀表达式中,直到遇到左括号
5. 如果是操作符,则判断其优先级与栈顶操作符的优先级,如果栈顶操作符优先级大于等于当前操作符,则弹出栈顶操作符并输出到后缀表达式中,然后重复此步骤;否则将当前操作符压入栈中
6. 扫描完毕后,如果栈中还有操作符,则依次弹出并输出到后缀表达式中
具体实现代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LEN 100
typedef struct Stack {
char data[MAX_LEN];
int top;
} Stack;
void initStack(Stack *s) {
s->top = -1;
}
void push(Stack *s, char c) {
if (s->top == MAX_LEN - 1) {
printf("Stack is full!\n");
exit(EXIT_FAILURE);
}
s->data[++(s->top)] = c;
}
char pop(Stack *s) {
if (s->top == -1) {
printf("Stack is empty!\n");
exit(EXIT_FAILURE);
}
return s->data[(s->top)--];
}
char peek(Stack *s) {
if (s->top == -1) {
printf("Stack is empty!\n");
exit(EXIT_FAILURE);
}
return s->data[s->top];
}
int isOperator(char c) {
return (c == '+' || c == '-' || c == '*' || c == '/');
}
int operatorPriority(char c) {
int priority = 0;
switch (c) {
case '+':
case '-':
priority = 1;
break;
case '*':
case '/':
priority = 2;
break;
default:
break;
}
return priority;
}
void infixToPostfix(char infix[], char postfix[]) {
Stack s;
initStack(&s);
int i, j = 0;
for (i = 0; infix[i] != '\0'; ++i) {
if (infix[i] == '(') {
push(&s, infix[i]);
} else if (infix[i] == ')') {
while (peek(&s) != '(') {
postfix[j++] = pop(&s);
}
pop(&s);
} else if (isOperator(infix[i])) {
while (s.top != -1 && operatorPriority(peek(&s)) >= operatorPriority(infix[i])) {
postfix[j++] = pop(&s);
}
push(&s, infix[i]);
} else {
postfix[j++] = infix[i];
}
}
while (s.top != -1) {
postfix[j++] = pop(&s);
}
postfix[j] = '\0';
}
int main() {
char infix[MAX_LEN];
char postfix[MAX_LEN];
printf("Please enter the infix expression:\n");
scanf("%s", infix);
infixToPostfix(infix, postfix);
printf("The postfix expression is: %s\n", postfix);
return 0;
}
```
阅读全文
相关推荐
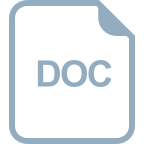
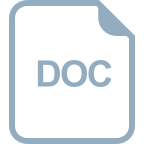
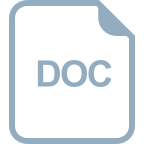
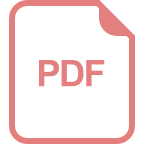











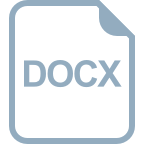
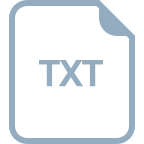

