请举例用代码实现上述要求
时间: 2024-05-06 22:15:52 浏览: 132
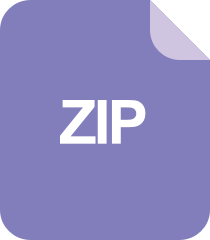
一个代码例子
以下是一个简单的示例代码,用于实现一个类似于 ChatGPT 的 AI 对话系统:
```python
import torch
from transformers import GPT2Tokenizer, GPT2LMHeadModel
class ChitGPT:
def __init__(self):
self.tokenizer = GPT2Tokenizer.from_pretrained('gpt2')
self.model = GPT2LMHeadModel.from_pretrained('gpt2')
self.device = torch.device('cuda' if torch.cuda.is_available() else 'cpu')
self.model.to(self.device)
self.model.eval()
def generate_response(self, prompt):
input_ids = self.tokenizer.encode(prompt, return_tensors='pt').to(self.device)
output = self.model.generate(input_ids, max_length=50, do_sample=True)
response = self.tokenizer.decode(output[0], skip_special_tokens=True)
return response
```
在上面的代码中,我们使用了 Hugging Face 提供的 transformers 库,该库提供了各种预训练的自然语言处理模型,包括 GPT-2。在 `ChitGPT` 类的初始化方法中,我们使用了 `GPT2Tokenizer` 和 `GPT2LMHeadModel` 类从预训练模型中加载 tokenizer 和模型。然后,我们将模型移动到 GPU 上(如果可用),并将其设置为评估模式。
在 `generate_response` 方法中,我们首先使用 tokenizer 将输入的文本编码为 token ids,然后将其转换为 PyTorch 张量并将其移动到 GPU 上。接下来,我们使用模型的 `generate` 方法来生成 AI 的响应,并将其解码为自然语言文本。最后,我们返回 AI 的响应。
阅读全文
相关推荐


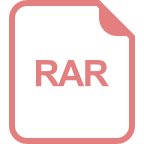












