用js写一个时间复杂度最少的排序
时间: 2024-05-06 18:20:15 浏览: 184
目前已知的时间复杂度最少的排序算法是基数排序(Radix Sort),时间复杂度为O(nk),其中n为数组长度,k为数组中最大元素的位数。
以下是使用JavaScript实现基数排序的代码:
```javascript
function radixSort(arr) {
const maxNum = Math.max(...arr);
const maxDigit = String(maxNum).length;
let buckets = Array.from({length: 10}, () => []);
for (let i = 0; i < maxDigit; i++) {
for (let j = 0; j < arr.length; j++) {
const digit = getDigit(arr[j], i);
buckets[digit].push(arr[j]);
}
arr = [].concat(...buckets);
buckets = Array.from({length: 10}, () => []);
}
return arr;
}
function getDigit(num, i) {
return Math.floor(Math.abs(num) / Math.pow(10, i)) % 10;
}
```
该算法首先找出数组中最大的数字,确定需要排序的位数,然后从最低位开始,将数字按照个位、十位、百位等进行排序,最终得到排好序的数组。
阅读全文
相关推荐
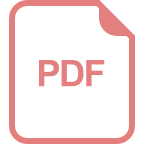
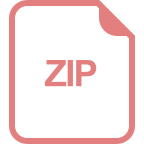
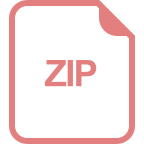
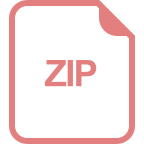
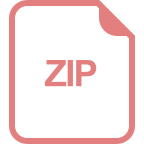
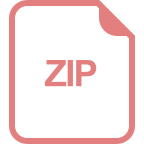
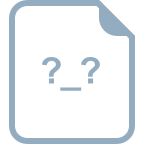
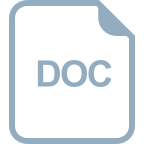
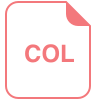
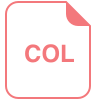
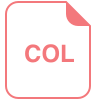
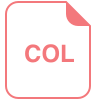
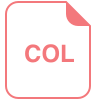
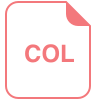
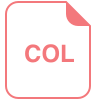
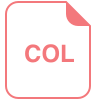
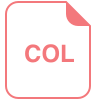