【链表性能分析】:打破JavaScript链表操作的常见误区
发布时间: 2024-09-14 10:27:25 阅读量: 61 订阅数: 29 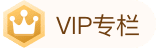
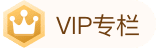
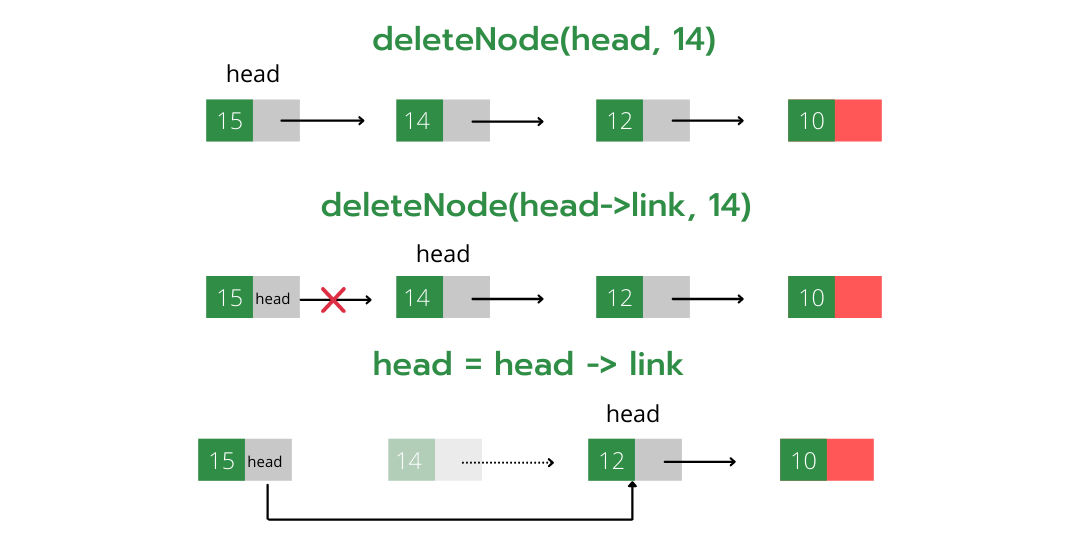
# 1. 链表基础与JavaScript实现
## 1.1 链表的基本概念
链表是一种常见的基础数据结构,其主要通过节点将数据和下一个节点的引用链接起来,形成一个线性结构。与数组相比,链表的优势在于动态分配内存,实现方便的插入和删除操作。
## 1.2 JavaScript中的链表实现
在JavaScript中实现链表并不复杂,通过构造函数来定义节点,其中包含数据和指向下一个节点的链接(next)。链表本身则由头节点(head)开始,形成链式结构。
```javascript
function ListNode(val) {
this.val = val;
this.next = null;
}
function LinkedList() {
this.head = null;
}
```
## 1.3 链表操作方法
链表的基本操作包括插入节点、删除节点、搜索节点等。在实现时,需要注意更新相关节点的指向关系,以保证链表的连贯性和正确性。
```javascript
// 插入节点
LinkedList.prototype.insert = function(val) {
let newNode = new ListNode(val);
newNode.next = this.head;
this.head = newNode;
};
// 删除节点
LinkedList.prototype.delete = function(val) {
let current = this.head,
previous = null;
while(current !== null && current.val !== val) {
previous = current;
current = current.next;
}
if(current === null) return;
if(previous === null) {
this.head = current.next;
} else {
previous.next = current.next;
}
};
```
通过上述的JavaScript代码示例,我们可以看到链表在内存管理和数据操作上具有灵活性。接下来的章节,我们将探讨链表操作的性能开销以及实际应用场景下的性能评估。
# 2. 链表操作的性能开销
## 2.1 时间复杂度分析
链表作为一种线性数据结构,其性能开销主要体现在时间复杂度上。时间复杂度用于衡量算法执行时间随输入数据量增长的变化趋势,是理解数据结构性能的重要指标。
### 2.1.1 插入操作的时间复杂度
在链表中进行插入操作时,我们通常关注的是在链表头部、尾部或者中间某个位置插入节点的效率。由于链表不需像数组那样移动元素,插入操作的时间复杂度通常为 O(1)。然而,若是在顺序插入时,为了找到特定位置,需要遍历链表,时间复杂度则为 O(n)。
```javascript
class ListNode {
constructor(value, next = null) {
this.value = value;
this.next = next;
}
}
function insertNodeAtBeginning(head, value) {
let newNode = new ListNode(value);
newNode.next = head;
return newNode;
}
// 示例代码:在链表头部插入节点
// 假设 head 是链表的头节点
head = insertNodeAtBeginning(head, newNodeValue);
```
执行逻辑说明:`insertNodeAtBeginning` 函数将创建一个新节点并将其设置为链表的新头部,这个操作只需要常数时间。
### 2.1.2 删除操作的时间复杂度
与插入类似,删除操作同样依赖于目标位置。删除链表头部节点的时间复杂度为 O(1),删除尾部节点需要遍历整个链表,时间复杂度为 O(n)。
```javascript
function removeNode(head, value) {
if (head === null) return null;
if (head.value === value) return head.next;
let current = head;
while (current.next !== null) {
if (current.next.value === value) {
current.next = current.next.next;
return head;
}
current = current.next;
}
return head;
}
// 示例代码:删除链表中的节点
head = removeNode(head, nodeToDeleteValue);
```
执行逻辑说明:`removeNode` 函数遍历链表,寻找值为 `value` 的节点并删除它。这个操作在最坏的情况下,即删除尾部节点时,需要遍历整个链表。
### 2.1.3 搜索操作的时间复杂度
在链表中进行搜索操作,如果需要从头到尾遍历链表,时间复杂度是 O(n)。
## 2.2 空间复杂度分析
空间复杂度考虑的是在实现数据结构时所需要的额外空间量。在分析链表的空间复杂度时,我们主要考虑的是节点分配的空间开销,以及由此可能引发的内存碎片化问题。
### 2.2.1 节点分配的空间开销
每个链表节点通常包含值和指针两个部分。值存储数据,指针指向下一个节点的位置。节点分配的总空间取决于数据的大小和指针的大小。
```plaintext
节点空间开销 = 数据空间 + 指针空间
```
### 2.2.2 内存碎片化问题
链表由于其动态分配特性,在频繁的插入和删除操作中,可能会造成内存碎片化,即内存中存在很多小的、不连续的空间。这会导致实际可用的内存空间大于分配的内存空间,影响系统的性能。
## 2.3 实际应用场景下的性能评估
在实际应用中,链表的性能往往与数组相比较。理解它们之间的性能差异对于选择合适的数据结构至关重要。
### 2.3.1 链表与数组的性能比较
数组使用连续的内存空间,访问任一元素的时间复杂度为 O(1)。相比之下,链表在插入和删除操作上可能更优,因为不需要移动大量元素。因此,如果频繁进行插入和删除操作,链表是更优的选择。
### 2.3.2 链表在JavaScript中的实际使用案例
在JavaScript中,由于其自动垃圾回收机制,链表操作通常比其他手动管理内存的编程语言更为简便。然而,由于其较低的内存访问速度和遍历效率,链表不如数组在实际中常用。
```javascript
// JavaScript 中使用链表的示例
let head = new ListNode(1);
head.next = new ListNode(2);
head.next.next = new ListNode(3);
// ... 进行链表操作 ...
```
代码块说明:上述代码展示了如何在JavaScript中创建一个简单的链表,并按顺序添加节点。实际使用时,链表结构使得访问特定节点需要遍历链表,这在数据量大时可能成为性能瓶颈。
# 3. 链表的常见误区与破解
## 3.1 误区一:链表总是比数组快
### 3.1.1 误区成因分析
在讨论链表和数组的性能时,常常会听到这样的说法:“链表操作比数组快,因为链表可以在任意位置进行插入和删除操作,而数组则需要移动元素以保持连续性。” 这种观念导致了链表比数组快的误区。实际上,这种说法并不总是正确的。在某些情况下,数组可能比链表更有效率,尤其是在现代的CPU缓存架构下。数组通过索引直接访问元素,时间复杂度为O(1),而链表则需要遍历节点来定位元素,时间复杂度为O(n)。
### 3.1.2 实验验证与结果解析
为了验证这一误区,我们可以设计一个简单的实验。在JavaScript环境中,我们可以使用Array和LinkedList的两种数据结构进行一系列的插入和删除操作,并记录它们所花费的时间。以
0
0
相关推荐
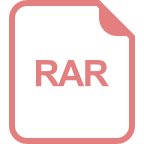
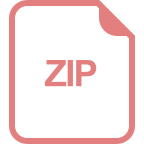
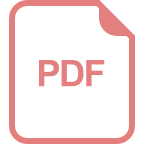
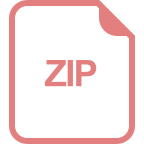
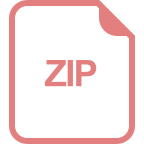
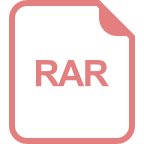
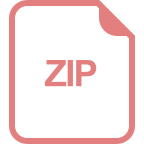