【链表并发挑战】:探索多线程环境下JavaScript链表的实现
发布时间: 2024-09-14 10:43:24 阅读量: 86 订阅数: 28 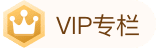
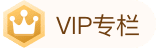
# 1. JavaScript中的链表基础知识
在数据结构的世界里,链表是一种基础而又强大的结构,尤其在JavaScript这样的动态语言中,链表的作用不可小觑。相比数组等其他线性结构,链表以其独特的节点存储方式,提供了高效的数据插入和删除操作。本章将从链表的定义开始,逐步带你了解它的基本操作和特点。
## 1.1 链表的定义
链表由一系列节点组成,每个节点包含数据和指向下一个节点的引用。链表的头节点称为链表的首,尾节点则没有指向下一个节点的引用,即它的下一个引用是null。根据节点间的链接方向,链表可以是单向的,也可以是双向的。
## 1.2 链表的基本操作
链表的核心操作主要包括插入节点、删除节点和遍历节点。在JavaScript中,因为没有内建的链表类型,我们需要自定义这些操作。例如,要在链表的末尾添加一个节点,我们可以:
```javascript
class ListNode {
constructor(value) {
this.value = value;
this.next = null;
}
}
class LinkedList {
constructor() {
this.head = null;
}
append(value) {
const newNode = new ListNode(value);
if (!this.head) {
this.head = newNode;
return;
}
let current = this.head;
while(current.next) {
current = current.next;
}
current.next = newNode;
}
}
let list = new LinkedList();
list.append(1);
list.append(2);
//链表现在是 1->2->null
```
本章通过简单的代码示例,让我们理解链表的基本结构和操作。接下来,我们将深入探讨链表如何在多线程环境中处理并发挑战。
# 2. 多线程环境下的并发挑战
在多线程编程的世界里,"并发"是一个关键词,它代表了程序执行的并行性,为计算机性能的提升带来了巨大的潜力。然而,随着线程数量的增加,实现线程安全的数据结构和算法,避免竞态条件和数据不一致,成为了一个严峻的挑战。尤其在JavaScript这类通常用于单线程环境的编程语言中,多线程编程引入了复杂性,但也提供了新的可能性。
### 3.1 锁的机制与应用
#### 3.1.1 互斥锁的原理
互斥锁是一种常见的同步机制,它确保在任何给定时刻,只有一个线程可以访问被锁保护的资源。这通过阻塞(或挂起)其它尝试访问该资源的线程来实现,直至锁被释放。互斥锁通过保证资源的独占访问,防止了数据竞争和其他并发问题。
在JavaScript中,虽然原生并不直接支持互斥锁,但可以通过使用Web Workers和共享内存,或者引入第三方库如`async`和`mutex-js`来实现类似机制。
```javascript
const { Mutex } = require('mutex-js');
async function criticalSection() {
const mutex = new Mutex();
await mutex.acquire();
try {
// 临界区代码,只有一个线程可以执行
} finally {
mutex.release();
}
}
```
#### 3.1.2 读写锁的实现
读写锁(也称为共享-独占锁)允许多个读操作同时进行,但写操作必须独占。这种锁特别适用于读操作远远多于写操作的场景,能够显著提高并发性能。
```javascript
class ReadWriteLock {
constructor() {
this.readers = 0;
this.writersWaiting = 0;
this.writeAccess = false;
this.readAccess = new Semaphore(0);
this.writeAccess = new Semaphore(1);
}
acquireRead() {
this.readAccess.acquire();
this.readers++;
if (this.writersWaiting > 0) {
this.readAccess.release();
} else {
this.readAccess.acquire();
}
}
releaseRead() {
this.readers--;
this.readAccess.release();
if (this.readers === 0 && this.writersWaiting > 0) {
this.writeAccess.release();
}
}
acquireWrite() {
this.writersWaiting++;
this.writeAccess.acquire();
this.writersWaiting--;
}
releaseWrite() {
this.writeAccess.release();
}
}
```
### 3.2 非阻塞同步技术
#### 3.2.1 原子操作的使用
原子操作是不可分割的操作,在执行过程中,不会被任何其他线程中断。现代JavaScript引擎提供了对原子操作的支持,例如`Atomics`对象,这是通过使用WebAssembly和底层CPU指令集实现的。
```javascript
const sharedArrayBuffer = new SharedArrayBuffer(1024);
const int32View = new Int32Array(sharedArrayBuffer);
Atomics.add(int32View, 0, 1); // 将索引0处的值加1
```
#### 3.2.2 无锁队列的构建
无锁数据结构设计得当的话,可以避免因线程阻塞带来的性能开销。无锁队列通过使用原子操作和特定的内存操作来避免锁的使用。
```javascript
class LockFreeQueue {
constructor() {
this.head = 0;
this.tail = 0;
this.queue = new Array(1024);
}
enqueue(value) {
const tail = Atomics.load(this.queue, this.tail);
Atomics.store(this.queue, tail, value);
Atomics.store(this.queue, this.tail, tail + 1);
}
dequeue() {
const head = Atomics.load(this.queue, this.head);
if (head !== Atomics.load(this.queue, this.tail)) {
return Atomics.load(this.queue, head);
}
return null;
}
}
```
### 3.3 内存模型与可见性
#### 3.3.1 JavaScript的内存模型
JavaScript内存模型定义了线程如何与共享内存交互,以及变量可见性的规则。理解JavaScript的内存模型对于编写正确的并发程序至关重要。
在JavaScript中,由于其单线程的本质,内存模型通常较为简单。然而,在Web Workers等多线程环境中,内存模型变得复杂。JavaScript通过事件循环和消息传递来维持线程间的交互。
#### 3.3.2 变量可见性问题与解决策略
在多线程环境中,变量可见性是必须关注的问题。变量修改可能不会立即对其他线程可见,可能导致意外的行为。
为了确保变量的可见性,JavaScript提供了`SharedArrayBuffer`和`Atomics`对象,通过使用这些特性,可以确保数据在多线程之间的一致性。
```javascript
const sharedArrayBuffer = new SharedArrayBuffer(1024);
const int32View = new Int32Array(sharedArrayBuffer);
Atomics.add(int32View, 0, 1); // 增加共享数组中索引0处的值
```
通过锁的机制、非阻塞同步技术以及对内存模型的理解,我们能够设计出既高效又稳定的多线程程序。在JavaScript中,尽管现代浏览器提供了越来越
0
0
相关推荐
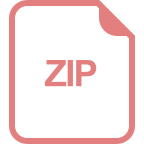
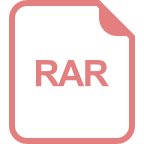
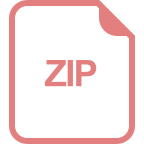
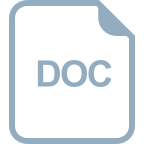
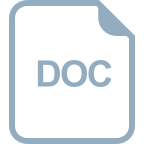
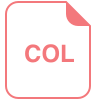
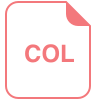
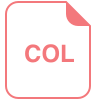
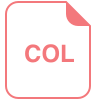