用C语言写一个五子棋小游戏,要求:实现人和AI电脑的对弈
时间: 2023-05-24 10:02:47 浏览: 86
A:
这里提供一个简单的五子棋小游戏的C语言代码示例,其中实现了人和AI电脑的对弈。请注意,该代码仅供参考,可能并非完美或最优解。
```c
#include <stdio.h>
#include <stdlib.h>
/* 定义棋盘大小为15x15 */
#define ROWS 15
#define COLS 15
/* 棋子颜色:BLACK为1,WHITE为2,EMPTY为0 */
#define BLACK 1
#define WHITE 2
#define EMPTY 0
/* 电脑AI的棋子颜色为黑色 */
#define AI_COLOR BLACK
/* 定义棋盘数组 */
int board[ROWS][COLS];
/* 判断是否胜利 */
int is_win(int x, int y, int color) {
int i, j, count;
/* 水平方向 */
count = 1;
for (j = y - 1; j >= 0 && board[x][j] == color; j--) {
count++;
}
for (j = y + 1; j < COLS && board[x][j] == color; j++) {
count++;
}
if (count >= 5) {
return 1; /* 胜利 */
}
/* 垂直方向 */
count = 1;
for (i = x - 1; i >= 0 && board[i][y] == color; i--) {
count++;
}
for (i = x + 1; i < ROWS && board[i][y] == color; i++) {
count++;
}
if (count >= 5) {
return 1; /* 胜利 */
}
/* 左上到右下斜方向 */
count = 1;
for (i = x - 1, j = y - 1; i >= 0 && j >= 0 && board[i][j] == color; i--, j--) {
count++;
}
for (i = x + 1, j = y + 1; i < ROWS && j < COLS && board[i][j] == color; i++, j++) {
count++;
}
if (count >= 5) {
return 1; /* 胜利 */
}
/* 左下到右上斜方向 */
count = 1;
for (i = x + 1, j = y - 1; i < ROWS && j >= 0 && board[i][j] == color; i++, j--) {
count++;
}
for (i = x - 1, j = y + 1; i >= 0 && j < COLS && board[i][j] == color; i--, j++) {
count++;
}
if (count >= 5) {
return 1; /* 胜利 */
}
return 0; /* 未胜利 */
}
/* 打印棋盘 */
void print_board() {
int i, j;
for (i = 0; i < ROWS; i++) {
for (j = 0; j < COLS; j++) {
if (board[i][j] == BLACK) {
printf("x ");
} else if (board[i][j] == WHITE) {
printf("o ");
} else {
printf(". ");
}
}
printf("\n");
}
}
/* 下黑子 */
void put_black(int x, int y) {
board[x][y] = BLACK;
}
/* 下白子 */
void put_white(int x, int y) {
board[x][y] = WHITE;
}
/* 判断是否为有效位置 */
int is_valid(int x, int y) {
if (x >= 0 && x < ROWS && y >= 0 && y < COLS && board[x][y] == EMPTY) {
return 1;
} else {
return 0;
}
}
/* 判断是否已下满 */
int is_full() {
int i, j;
for (i = 0; i < ROWS; i++) {
for (j = 0; j < COLS; j++) {
if (board[i][j] == EMPTY) {
return 0;
}
}
}
return 1; /* 已满 */
}
/* 电脑AI下棋的方法 */
void ai_turn() {
int i, j, k, l, x = -1, y = -1, count_max = 0, count;
/* 找到权值最大的位置 */
for (i = 0; i < ROWS; i++) {
for (j = 0; j < COLS; j++) {
if (board[i][j] == EMPTY) {
count = 0;
for (k = i - 1; k <= i + 1; k++) {
for (l = j - 1; l <= j + 1; l++) {
if (k >= 0 && k < ROWS && l >= 0 && l < COLS && board[k][l] == AI_COLOR) {
count++;
}
}
}
if (count >= count_max) {
count_max = count;
x = i;
y = j;
}
}
}
}
put_black(x, y);
printf("Computer puts black at (%d, %d).\n", x, y);
}
int main() {
int x, y;
/* 初始化棋盘 */
int i, j;
for (i = 0; i < ROWS; i++) {
for (j = 0; j < COLS; j++) {
board[i][j] = EMPTY;
}
}
/* 主循环 */
while (1) {
/* 玩家下棋 */
printf("Please enter the coordinate (x y) to put white (e.g. 3 4): ");
scanf("%d %d", &x, &y);
if (!is_valid(x, y)) {
printf("Invalid coordinate.\n");
continue;
}
put_white(x, y);
print_board();
if (is_win(x, y, WHITE)) {
printf("You win!\n");
break;
}
if (is_full()) {
printf("Draw.\n");
break;
}
/* 电脑AI下棋 */
ai_turn();
print_board();
if (is_win(x, y, BLACK)) {
printf("Computer wins!\n");
break;
}
if (is_full()) {
printf("Draw.\n");
break;
}
}
return 0;
}
```
该代码实现了基本的五子棋人机对战,但是AI的实现只是通过简单的权值计算选择落子位置,没有过多考虑人类下棋的策略,如果需要进行五子棋的更深层次开发,还需要不断优化完善算法和策略。
相关推荐
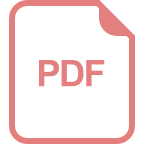
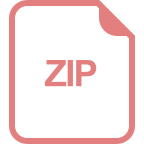
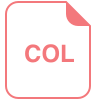
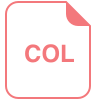
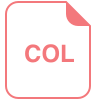
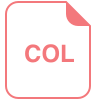
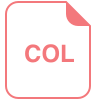









