ros用python让turtlesim画出一个五角星的轨迹的程序
时间: 2024-05-02 12:19:53 浏览: 15
Sure, here's a Python script that uses ROS to make Turtlesim draw a five-pointed star:
```
#!/usr/bin/env python
import rospy
from geometry_msgs.msg import Twist
def draw_star():
rospy.init_node('draw_star', anonymous=True)
pub = rospy.Publisher('/turtle1/cmd_vel', Twist, queue_size=10)
rate = rospy.Rate(1)
move_cmd = Twist()
# Move forward
move_cmd.linear.x = 1
pub.publish(move_cmd)
rospy.sleep(2)
# Turn left
move_cmd.linear.x = 0
move_cmd.angular.z = 1
pub.publish(move_cmd)
rospy.sleep(2)
# Move forward
move_cmd.linear.x = 1
move_cmd.angular.z = 0
pub.publish(move_cmd)
rospy.sleep(2)
# Turn right
move_cmd.linear.x = 0
move_cmd.angular.z = -1
pub.publish(move_cmd)
rospy.sleep(2)
# Move forward
move_cmd.linear.x = 1
move_cmd.angular.z = 0
pub.publish(move_cmd)
rospy.sleep(2)
# Turn left
move_cmd.linear.x = 0
move_cmd.angular.z = 1
pub.publish(move_cmd)
rospy.sleep(2)
# Move forward
move_cmd.linear.x = 1
move_cmd.angular.z = 0
pub.publish(move_cmd)
rospy.sleep(2)
# Turn right
move_cmd.linear.x = 0
move_cmd.angular.z = -1
pub.publish(move_cmd)
rospy.sleep(2)
# Move forward
move_cmd.linear.x = 1
move_cmd.angular.z = 0
pub.publish(move_cmd)
rospy.sleep(2)
# Turn left
move_cmd.linear.x = 0
move_cmd.angular.z = 1
pub.publish(move_cmd)
rospy.sleep(2)
# Stop moving
move_cmd.linear.x = 0
move_cmd.angular.z = 0
pub.publish(move_cmd)
if __name__ == '__main__':
try:
draw_star()
except rospy.ROSInterruptException:
pass
```
This script moves the turtle forward for a few seconds, then turns left, moves forward again, turns right, moves forward, turns left, moves forward, turns right, moves forward, turns left, and finally stops moving. The result is a five-pointed star.
相关推荐
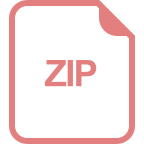
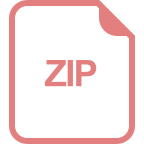














